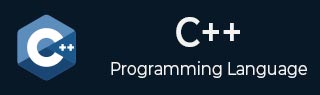
- C++ Home
- C++ Overview
- C++ Environment Setup
- C++ Basic Syntax
- C++ Comments
- C++ Hello World
- C++ Omitting Namespace
- C++ Tokens
- C++ Constants/Literals
- C++ Keywords
- C++ Identifiers
- C++ Data Types
- C++ Numeric Data Types
- C++ Character Data Type
- C++ Boolean Data Type
- C++ Variable Types
- C++ Variable Scope
- C++ Multiple Variables
- C++ Basic Input/Output
- C++ Modifier Types
- C++ Storage Classes
- C++ Numbers
- C++ Enumeration
- C++ Enum Class
- C++ References
- C++ Date & Time
- C++ Operators
- C++ Arithmetic Operators
- C++ Relational Operators
- C++ Logical Operators
- C++ Bitwise Operators
- C++ Assignment Operators
- C++ sizeof Operator
- C++ Conditional Operator
- C++ Comma Operator
- C++ Member Operators
- C++ Casting Operators
- C++ Pointer Operators
- C++ Operators Precedence
- C++ Unary Operators
- C++ Control Statements
- C++ Decision Making
- C++ if Statement
- C++ if else Statement
- C++ Nested if Statements
- C++ switch Statement
- C++ Nested switch Statements
- C++ Loop Types
- C++ while Loop
- C++ for Loop
- C++ do while Loop
- C++ Foreach Loop
- C++ Nested Loops
- C++ break Statement
- C++ continue Statement
- C++ goto Statement
- C++ Strings
- C++ Strings
- C++ Loop Through a String
- C++ String Length
- C++ String Concatenation
- C++ String Comparison
- C++ Functions
- C++ Functions
- C++ Multiple Function Parameters
- C++ Recursive Function
- C++ Return Values
- C++ Function Overloading
- C++ Function Overriding
- C++ Default Arguments
- C++ Arrays
- C++ Arrays
- C++ Multidimensional Arrays
- C++ Pointer to an Array
- C++ Passing Arrays to Functions
- C++ Return Array from Functions
- C++ Structure & Union
- C++ Structures
- C++ Unions
- C++ Pointers
- C++ Pointers
- C++ Dereferencing
- C++ Modify Pointers
- C++ Class and Objects
- C++ Object Oriented
- C++ Classes & Objects
- C++ Class Member Functions
- C++ Class Access Modifiers
- C++ Static Class Members
- C++ Static Data Members
- C++ Static Member Function
- C++ Inline Functions
- C++ this Pointer
- C++ Friend Functions
- C++ Pointer to Classes
- C++ Constructors
- C++ Constructor & Destructor
- C++ Default Constructors
- C++ Parameterized Constructors
- C++ Copy Constructor
- C++ Constructor Overloading
- C++ Constructor with Default Arguments
- C++ Delegating Constructors
- C++ Constructor Initialization List
- C++ Dynamic Initialization Using Constructors
- C++ Object-oriented
- C++ Overloading
- C++ Polymorphism
- C++ Abstraction
- C++ Encapsulation
- C++ Interfaces
- C++ Virtual Function
- C++ Pure Virtual Functions & Abstract Classes
- C++ File Handling
- C++ Files and Streams
- C++ Reading From File
- C++ Advanced
- C++ Exception Handling
- C++ Dynamic Memory
- C++ Namespaces
- C++ Templates
- C++ Preprocessor
- C++ Signal Handling
- C++ Multithreading
- C++ Web Programming
- C++ Socket Programming
- C++ Concurrency
- C++ Advanced Concepts
- C++ Lambda Expression
- C++ unordered_multiset
C++ Strings
C++ provides following two types of string representations −
- The C-style character string.
- The string class type introduced with Standard C++.
The C-Style Character String
The C-style character string originated within the C language and continues to be supported within C++. This string is actually a one-dimensional array of characters which is terminated by a null character '\0'. Thus a null-terminated string contains the characters that comprise the string followed by a null.
The following declaration and initialization create a string consisting of the word "Hello". To hold the null character at the end of the array, the size of the character array containing the string is one more than the number of characters in the word "Hello."
char greeting[6] = {'H', 'e', 'l', 'l', 'o', '\0'};
If you follow the rule of array initialization, then you can write the above statement as follows −
char greeting[] = "Hello";
Following is the memory presentation of above defined string in C/C++ −
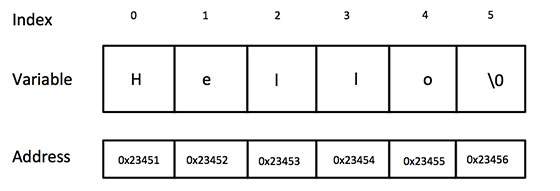
Actually, you do not place the null character at the end of a string constant. The C++ compiler automatically places the '\0' at the end of the string when it initializes the array. Let us try to print above-mentioned string −
Example
#include <iostream> using namespace std; int main () { char greeting[6] = {'H', 'e', 'l', 'l', 'o', '\0'}; cout << "Greeting message: "; cout << greeting << endl; return 0; }
When the above code is compiled and executed, it produces the following result −
Greeting message: Hello
C Style String Functions
C++ supports a wide range of functions that manipulate null-terminated strings. These functions are defined in <string.h> header file.
Sr.No | Function & Purpose |
---|---|
1 | Copies string s2 into string s1. |
2 | Concatenates string s2 onto the end of string s1. |
3 | Returns the length of string s1. |
4 | Returns 0 if s1 and s2 are the same; less than 0 if s1<s2; greater than 0 if s1>s2. |
5 | Returns a pointer to the first occurrence of character ch in string s1. |
6 | Returns a pointer to the first occurrence of string s2 in string s1. |
Example
Following example makes use of few of the above-mentioned functions −
#include <iostream> #include <cstring> using namespace std; int main () { char str1[10] = "Hello"; char str2[10] = "World"; char str3[10]; int len ; // copy str1 into str3 strcpy( str3, str1); cout << "strcpy( str3, str1) : " << str3 << endl; // concatenates str1 and str2 strcat( str1, str2); cout << "strcat( str1, str2): " << str1 << endl; // total lenghth of str1 after concatenation len = strlen(str1); cout << "strlen(str1) : " << len << endl; return 0; }
When the above code is compiled and executed, it produces result something as follows −
strcpy( str3, str1) : Hello strcat( str1, str2): HelloWorld strlen(str1) : 10
The String Class in C++
The standard C++ library provides a string class type that supports all the operations mentioned above, additionally much more functionality.
Example of String Class
Let us check the following example −
#include <iostream> #include <string> using namespace std; int main () { string str1 = "Hello"; string str2 = "World"; string str3; int len ; // copy str1 into str3 str3 = str1; cout << "str3 : " << str3 << endl; // concatenates str1 and str2 str3 = str1 + str2; cout << "str1 + str2 : " << str3 << endl; // total length of str3 after concatenation len = str3.size(); cout << "str3.size() : " << len << endl; return 0; }
When the above code is compiled and executed, it produces result something as follows −
str3 : Hello str1 + str2 : HelloWorld str3.size() : 10
Creating a String
We can create string variables in two ways, using either of the following methods −
Creating String Using Character Arrays
We can declare strings using the C-type arrays in the format of characters. This is done using the following syntax −
Syntax
char variable_name[len_value] ;
Here, len_value is the length of the character array.
Example of Creating String using Character Array
In the following examples, we are declaring a character array, and assigning values to it.
#include <iostream> using namespace std; int main() { char s[5]={'h','e','l','l','o'}; cout<<s<<endl; return 0; }
Output
hello
Creating String using <string>
We can declare a String variable using the 'string' keyword. This is included in the <string> header file. The syntax of declaring a string is explained as follows −
Syntax
string variable_name = [value];
Here, [value] is an optional and can be used to assign value during the declaration.
Example
In the following examples, we are declaring a string variable, assigning a value to it.
#include <iostream> using namespace std; int main() { string s="a merry tale"; cout<<s; return 0; }
Output
a merry tale
Traversing a String (Iterate Over a String)
We can iterate over a string in two ways −
Using looping statements
We can traverse a string using for loops, while loops and do while loops using a pointer to the first and the last index in the string.
Using iterators
Using range based loops, we can iterate over the string using iterators. This is achieved using ":" operator while running a range based loop.
Example of Iterating a String
The following example code shows string traversal using both of these methods −
#include <iostream> using namespace std; int main() { string s="Hey, I am at TP."; for(int i=0;i<s.length();i++){ cout<<s[i]<<" "; } cout<<endl; for(char c:s){ cout<<c<<" "; } return 0; }
Output
H e y , I a m a t T P . H e y , I a m a t T P .
Accessing Characters of String
We can access the characters of a string using both iterators and pointer to the indices of the string.
Example
The following example code shows how we can access the characters in a string −
#include <iostream> using namespace std; int main() { string s="Hey, I am at TP."; cout<<s<<endl; for(int i=0;i<s.length();i++){ s[i]='A'; } cout<<s<<endl; for(char &c:s){ c='B'; } cout<<s<<endl; return 0; }
Output
Hey, I am at TP. AAAAAAAAAAAAAAAA BBBBBBBBBBBBBBBB
String Functions
String is an object of the <string> class, and hence, it has a variety of functions that users can utilize for a variety of operations. Some of these functions are as follows −
Function | Description |
---|---|
length() | This function returns the length of the string. |
swap() | This function is used to swap the values of 2 strings. |
size() | Used to find the size of string |
resize() | This function is used to resize the length of the string up to the given number of characters. |
find() | Used to find the string which is passed in parameters |
push_back() | This function is used to push the character at the end of the string |
pop_back() | This function is used to pop the last character from the string |
clear() | This function is used to remove all the elements of the string. |
find() | This function is used to search for a certain substring inside a string and returns the position of the first character of the substring. |
replace() | This function is used to replace each element in the range [first, last) that is equal to old value with new value. |
substr() | This function is used to create a substring from a given string. |
compare() | This function is used to compare two strings and returns the result in the form of an integer. |
erase() | This function is used to remove a certain part of a string. |
Length of a String
The length of a string is the number of characters present in the string. Hence, the string "apple" has a length of 5 characters and the string "hello son" has a length of 9 characters (including empty spaces). This can be accessed using the length() method in <string> header file.
Syntax
The syntax is explained as follows −
int len = string_1.length();
Example
#include <iostream> #include <string> using namespace std; int main() { string x="hey boy"; cout<<x.length()<<endl; return 0; }
Output
7
String Concatenation
String concatenation is a way to add two strings together. This can be done using two ways −
Addition Operator
The addition operator is used to add two elements. In case of strings, the addition operator concatenates the two strings. This is clearly explained in the following example −
Example
#include <iostream> #include <string> using namespace std; int main() { string x = "10"; string y = "20"; cout<<x+y<<endl; return 0; }
Output
1020
This is different from integer addition. When we take two integers and add them using addition operator, we get the sum of the two numbers instead.
This is clearly explained in the following example −
Example
#include <iostream> #include <string> using namespace std; int main() { int x = 10; int y = 20; cout<<x+y<<endl; return 0; }
Output
30
Using string append() method
C++ is an object oriented programming language, and hence a string is actually an object, which contain functions that can perform certain operations on strings. We can use string append() method to append one string to another.
The syntax of this operation is as follows −
Syntax
string_1.append(string_2);
The usage of this method is depicted clearly in the following example −
Example
#include <iostream> #include <string> using namespace std; int main() { string x="hey boy"; string y=" now"; x.append(y); cout<<x<<endl; return 0; }
Output
hey boy now
String Input in C++
Strings can be taken as an input to the program, and the most common way to do this is by using cin method.
There are three methods to take a string as an input, and these methods are given as follows −
- cin
- getline()
- stringstream
Using cin Method
This is the simplest way to take a string as an input. The syntax is given as follows −
Syntax
cin>>string_name;
Example
#include <iostream> using namespace std; int main() { string s; cout << "Enter custom string : "<<endl; //enter the string here cin>>s; cout<<"The string is : "<<s; }
Using getline() Method
The getline() method can be used to read a string from an input stream. This is defined in the <string> header file. The syntax of the above method is given as follows −
Syntax
getline(cin, string_name);
Example
#include <iostream> using namespace std; int main() { string s; cout << "Enter String as input : " << endl; getline(cin, s); //enter the string here cout << "Printed string is : " << s << endl; return 0; }
Using stringstream
The stringstream class is used to take multiple strings as input, all at once. The syntax of the above method is given as follows −
Syntax
Stringstream object_name(string_name);
Example
#include <iostream> #include <sstream> #include <string> using namespace std; int main() { string s = "Hey, I am at TP"; stringstream object(s); string newstr; // >> operator will read from the stringstream object while (object >> newstr) { cout << newstr << " "; } return 0; }
Output
Hey, I am at TP