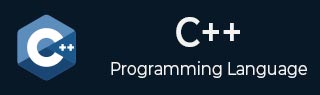
- C++ Home
- C++ Overview
- C++ Environment Setup
- C++ Basic Syntax
- C++ Comments
- C++ Hello World
- C++ Omitting Namespace
- C++ Tokens
- C++ Constants/Literals
- C++ Keywords
- C++ Identifiers
- C++ Data Types
- C++ Numeric Data Types
- C++ Character Data Type
- C++ Boolean Data Type
- C++ Variable Types
- C++ Variable Scope
- C++ Multiple Variables
- C++ Basic Input/Output
- C++ Modifier Types
- C++ Storage Classes
- C++ Numbers
- C++ Enumeration
- C++ Enum Class
- C++ References
- C++ Date & Time
- C++ Operators
- C++ Arithmetic Operators
- C++ Relational Operators
- C++ Logical Operators
- C++ Bitwise Operators
- C++ Assignment Operators
- C++ sizeof Operator
- C++ Conditional Operator
- C++ Comma Operator
- C++ Member Operators
- C++ Casting Operators
- C++ Pointer Operators
- C++ Operators Precedence
- C++ Unary Operators
- C++ Control Statements
- C++ Decision Making
- C++ if Statement
- C++ if else Statement
- C++ Nested if Statements
- C++ switch Statement
- C++ Nested switch Statements
- C++ Loop Types
- C++ while Loop
- C++ for Loop
- C++ do while Loop
- C++ Foreach Loop
- C++ Nested Loops
- C++ break Statement
- C++ continue Statement
- C++ goto Statement
- C++ Strings
- C++ Strings
- C++ Loop Through a String
- C++ String Length
- C++ String Concatenation
- C++ String Comparison
- C++ Functions
- C++ Functions
- C++ Multiple Function Parameters
- C++ Recursive Function
- C++ Return Values
- C++ Function Overloading
- C++ Function Overriding
- C++ Default Arguments
- C++ Arrays
- C++ Arrays
- C++ Multidimensional Arrays
- C++ Pointer to an Array
- C++ Passing Arrays to Functions
- C++ Return Array from Functions
- C++ Structure & Union
- C++ Structures
- C++ Unions
- C++ Pointers
- C++ Pointers
- C++ Dereferencing
- C++ Modify Pointers
- C++ Class and Objects
- C++ Object Oriented
- C++ Classes & Objects
- C++ Class Member Functions
- C++ Class Access Modifiers
- C++ Static Class Members
- C++ Static Data Members
- C++ Static Member Function
- C++ Inline Functions
- C++ this Pointer
- C++ Friend Functions
- C++ Pointer to Classes
- C++ Constructors
- C++ Constructor & Destructor
- C++ Default Constructors
- C++ Parameterized Constructors
- C++ Copy Constructor
- C++ Constructor Overloading
- C++ Constructor with Default Arguments
- C++ Delegating Constructors
- C++ Constructor Initialization List
- C++ Dynamic Initialization Using Constructors
- C++ Object-oriented
- C++ Overloading
- C++ Polymorphism
- C++ Abstraction
- C++ Encapsulation
- C++ Interfaces
- C++ Virtual Function
- C++ Pure Virtual Functions & Abstract Classes
- C++ File Handling
- C++ Files and Streams
- C++ Reading From File
- C++ Advanced
- C++ Exception Handling
- C++ Dynamic Memory
- C++ Namespaces
- C++ Templates
- C++ Preprocessor
- C++ Signal Handling
- C++ Multithreading
- C++ Web Programming
- C++ Socket Programming
- C++ Concurrency
- C++ Advanced Concepts
- C++ Lambda Expression
- C++ unordered_multiset
C++ Identifiers
C++ identifiers are unique names assigned to identify variables, functions, classes, arrays, and other user-defined items in a program.
The examples of identifier in C++ are −
int number = 10; string name = "John";
Here, number and name are identifiers for an integer and a string variable respectively.
Rules for Identifiers
- It must begin with a letter (uppercase "A-Z" or lowercase "a-z") or an underscore (_) but cannot start with a digit.
- After the first character, subsequent characters can be letters, digits (0-9), or underscores.
- Identifiers are case-sensitive (myVar and myvar are different).
- It cannot be a keyword (reserved word in C++), for example, int, bool, return, and while, etc.
- It must be unique within their namespace.
- Use meaningful names that reflect the purpose of the identifier (e.g, totalCount, calculateArea).
- In common practices, generally use camelCase or snake_case for readability.
- There is generally no strict limit on the length, but avoid long names as they make code harder to read and understand.
Types of Identifiers
Here are examples of identifiers in various cases −
1. Variable Identifiers
Variable identifiers are names given to variables in programming languages, which are used to identify reference data stored in those variables.
Here are a few examples of valid identifiers −
int age; // 'age' is an identifier for an integer variable double salary; // 'salary' is an identifier for a double variable char initial_alpha; // 'initial_alpha' is an identifier for a character variable
2. Constant Identifiers
Constant identifiers are names assigned to constants in programming, which represent fixed values that cannot be changed during the execution of a program.
Here is a simple example of a valid constant identifier −
const int MAX_SIZE = 100; // 'MAX_SIZE' is an identifier for a constant
3. Function Identifiers
Function identifiers are names assigned to functions in programming, which allow developers to define and call reusable blocks of code.
Some of the valid function identifiers are as follows −
void calculateSum() { // 'calculateSum' is an identifier for a function // function implementation } int getValue() { // 'getValue' is another function identifier return 42; }
4. Class Identifiers
Class identifiers are names assigned to classes in object-oriented programming which is used to define new data types that encapsulate attributes and behaviors associated with specific entities.
Here is a simple example of a valid class identifier −
class Person { // 'Person' is an identifier for a class public: int age; string name; };
Example of Invalid Identifiers
Here are a few examples of invalid identifiers −
- 2ndValue (as it starts with a digit)
- first-name (it contains a hyphen)
- @username (begins with a special character)
- my variable (contains a space)
- float (uses a reserved keyword)