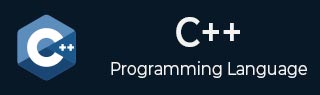
- C++ Home
- C++ Overview
- C++ Environment Setup
- C++ Basic Syntax
- C++ Comments
- C++ Hello World
- C++ Omitting Namespace
- C++ Tokens
- C++ Constants/Literals
- C++ Keywords
- C++ Identifiers
- C++ Data Types
- C++ Numeric Data Types
- C++ Character Data Type
- C++ Boolean Data Type
- C++ Variable Types
- C++ Variable Scope
- C++ Multiple Variables
- C++ Basic Input/Output
- C++ Modifier Types
- C++ Storage Classes
- C++ Numbers
- C++ Enumeration
- C++ Enum Class
- C++ References
- C++ Date & Time
- C++ Operators
- C++ Arithmetic Operators
- C++ Relational Operators
- C++ Logical Operators
- C++ Bitwise Operators
- C++ Assignment Operators
- C++ sizeof Operator
- C++ Conditional Operator
- C++ Comma Operator
- C++ Member Operators
- C++ Casting Operators
- C++ Pointer Operators
- C++ Operators Precedence
- C++ Unary Operators
- C++ Control Statements
- C++ Decision Making
- C++ if Statement
- C++ if else Statement
- C++ Nested if Statements
- C++ switch Statement
- C++ Nested switch Statements
- C++ Loop Types
- C++ while Loop
- C++ for Loop
- C++ do while Loop
- C++ Foreach Loop
- C++ Nested Loops
- C++ break Statement
- C++ continue Statement
- C++ goto Statement
- C++ Strings
- C++ Strings
- C++ Loop Through a String
- C++ String Length
- C++ String Concatenation
- C++ String Comparison
- C++ Functions
- C++ Functions
- C++ Multiple Function Parameters
- C++ Recursive Function
- C++ Return Values
- C++ Function Overloading
- C++ Function Overriding
- C++ Default Arguments
- C++ Arrays
- C++ Arrays
- C++ Multidimensional Arrays
- C++ Pointer to an Array
- C++ Passing Arrays to Functions
- C++ Return Array from Functions
- C++ Structure & Union
- C++ Structures
- C++ Unions
- C++ Pointers
- C++ Pointers
- C++ Dereferencing
- C++ Modify Pointers
- C++ Class and Objects
- C++ Object Oriented
- C++ Classes & Objects
- C++ Class Member Functions
- C++ Class Access Modifiers
- C++ Static Class Members
- C++ Static Data Members
- C++ Static Member Function
- C++ Inline Functions
- C++ this Pointer
- C++ Friend Functions
- C++ Pointer to Classes
- C++ Constructors
- C++ Constructor & Destructor
- C++ Default Constructors
- C++ Parameterized Constructors
- C++ Copy Constructor
- C++ Constructor Overloading
- C++ Constructor with Default Arguments
- C++ Delegating Constructors
- C++ Constructor Initialization List
- C++ Dynamic Initialization Using Constructors
- C++ Object-oriented
- C++ Overloading
- C++ Polymorphism
- C++ Abstraction
- C++ Encapsulation
- C++ Interfaces
- C++ Virtual Function
- C++ Pure Virtual Functions & Abstract Classes
- C++ File Handling
- C++ Files and Streams
- C++ Reading From File
- C++ Advanced
- C++ Exception Handling
- C++ Dynamic Memory
- C++ Namespaces
- C++ Templates
- C++ Preprocessor
- C++ Signal Handling
- C++ Multithreading
- C++ Web Programming
- C++ Socket Programming
- C++ Concurrency
- C++ Advanced Concepts
- C++ Lambda Expression
- C++ unordered_multiset
C++ Relational Operators
Relational Operators
In C++, relational operators are used to compare values or expressions. These check the relationship between the operands and return a result in a boolean (true or false).
These comparisons are based on conditions like equality, inequality, greater than, less than, etc.
Relational operators are a fundamental part of a programming language as they help in decision-making, loops, and conditional checks.
List of Relational Operators
This is the following list of the relational operators in C++ −
- Equal to (==) − It checks if two values are equal.
- Not equal to (!=) − It checks if two values are not equal.
- Greater than (>) − It checks if the left operand is greater than the right.
- Less than (<) − It checks if the left operand is less than the right.
- Greater than or equal to (>=) − It checks if the left operand is greater than or equal to the right.
- Less than or equal to (<=) − It checks if the left operand is less than or equal to the right.
Relational Operators Usage
Relational operators are used to compare two values or objects in C++. Here we will see the list of following, where relational operators can be used.
- Comparing Integers − It can be used for comparing integer data types like int, long, short, etc.
- Comparing Floating-Point Numbers − Relational operators can also be used to compare floating-point numbers (float, double, etc.). However, due to precision issues with floating-point arithmetic, the results may not always be as expected when dealing with very small or very large numbers.
- Comparing Characters and Strings − Relational operators compare characters based on their ASCII values. Strings are objects of the std::string class, and relational operators are overloaded to compare them lexicographically (in alphabetical order).
- Comparing Objects (Custom Classes) − In C++, you can overload relational operators for custom objects, allowing you to compare instances of a class based on certain criteria.
Example of Relational Operators
The following is an example of relational operators:
#include <iostream> #include <cmath> // For floating-point comparison #include <string> using namespace std; // Custom class to overload relational operators class Point { public: int x, y; Point(int x, int y) : x(x), y(y) {} bool operator>(const Point& p) { return (x*x + y*y) > (p.x*p.x + p.y*p.y); } bool operator==(const Point& p) { return (x == p.x && y == p.y); } }; int main() { // Comparing Integers int a = 5, b = 10; cout << (a > b) << " " << (a == b) << endl; // Comparing Floating-Point Numbers double x = 5.0, y = 5.0000001; cout << (fabs(x - y) < 1e-6) << endl; // Comparing Characters char c1 = 'A', c2 = 'B'; cout << (c1 < c2) << " " << (c1 == c2) << endl; // Comparing Strings string str1 = "apple", str2 = "banana"; cout << (str1 < str2) << " " << (str1 == str2) << endl; // Comparing Objects Point p1(3, 4), p2(5, 12); cout << (p1 > p2) << " " << (p1 == p2) << endl; return 0; }
Output
0 0 1 1 0 1 0 0 0
Relational Operators and Conditional Statements
In C++, Relational Operators in conditional statements help the program with decision-making and give results (true or false) based on the result of the comparison.
Syntax for if-else with Relational Operators
Here we will see the syntax for if-else with relational operators.
if (a > b) { // a is greater than b } else if (a == b) { // a is equal to b } else { // a is less than b }
Syntax for while Loop with Relational Operators
Here we will see the syntax for while loop with relational operators.
int i = 1; while (i <= 5) { if (i < 5) { // i is less than 5 } else if (i == 5) { // i is equal to 5 } i++; }
Relational Operators with Logical Operators
In C++, relational operators (>, <, ==, !=, >=, <=) can be combined with logical operators (&&, ||, !) to form complex expressions, which allow for more advanced decision-making. this is helpful when you need to check multiple conditions in one expression.
Example
#include <iostream> using namespace std; int main() { int age = 25, height = 180; // Check if age is between 18 and 30 and height is greater than 170 if (age >= 18 && age <= 30 && height > 170) cout << "The person qualifies!" << endl; // Check if age is outside 18-30 or height is <= 170 if (age < 18 || age > 30 || height <= 170) cout << "The person does not qualify!" << endl; // Check if age is NOT between 18 and 30 or height is NOT > 170 if (!(age >= 18 && age <= 30) || !(height > 170)) cout << "The person does not qualify due to NOT condition!" << endl; return 0; }
Output
The person qualifies!
Limitations of Relational Operators
- Precision issues with floating-point comparisons (e.g., float or double types may not behave as expected due to rounding errors).
- Comparing non-numeric types (e.g., comparing objects, strings, or custom classes might need custom operator overloads).
- Ambiguities in complex expressions where the order of evaluation or operator precedence can lead to unintended results.
- Type coercion when using relational operators with mixed data types (e.g., comparing int and double).