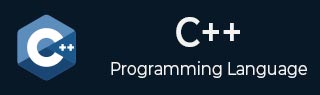
- C++ Home
- C++ Overview
- C++ Environment Setup
- C++ Basic Syntax
- C++ Comments
- C++ Hello World
- C++ Omitting Namespace
- C++ Tokens
- C++ Constants/Literals
- C++ Keywords
- C++ Identifiers
- C++ Data Types
- C++ Numeric Data Types
- C++ Character Data Type
- C++ Boolean Data Type
- C++ Variable Types
- C++ Variable Scope
- C++ Multiple Variables
- C++ Basic Input/Output
- C++ Modifier Types
- C++ Storage Classes
- C++ Numbers
- C++ Enumeration
- C++ Enum Class
- C++ References
- C++ Date & Time
- C++ Operators
- C++ Arithmetic Operators
- C++ Relational Operators
- C++ Logical Operators
- C++ Bitwise Operators
- C++ Assignment Operators
- C++ sizeof Operator
- C++ Conditional Operator
- C++ Comma Operator
- C++ Member Operators
- C++ Casting Operators
- C++ Pointer Operators
- C++ Operators Precedence
- C++ Unary Operators
- C++ Control Statements
- C++ Decision Making
- C++ if Statement
- C++ if else Statement
- C++ Nested if Statements
- C++ switch Statement
- C++ Nested switch Statements
- C++ Loop Types
- C++ while Loop
- C++ for Loop
- C++ do while Loop
- C++ Foreach Loop
- C++ Nested Loops
- C++ break Statement
- C++ continue Statement
- C++ goto Statement
- C++ Strings
- C++ Strings
- C++ Loop Through a String
- C++ String Length
- C++ String Concatenation
- C++ String Comparison
- C++ Functions
- C++ Functions
- C++ Multiple Function Parameters
- C++ Recursive Function
- C++ Return Values
- C++ Function Overloading
- C++ Function Overriding
- C++ Default Arguments
- C++ Arrays
- C++ Arrays
- C++ Multidimensional Arrays
- C++ Pointer to an Array
- C++ Passing Arrays to Functions
- C++ Return Array from Functions
- C++ Structure & Union
- C++ Structures
- C++ Unions
- C++ Pointers
- C++ Pointers
- C++ Dereferencing
- C++ Modify Pointers
- C++ Class and Objects
- C++ Object Oriented
- C++ Classes & Objects
- C++ Class Member Functions
- C++ Class Access Modifiers
- C++ Static Class Members
- C++ Static Data Members
- C++ Static Member Function
- C++ Inline Functions
- C++ this Pointer
- C++ Friend Functions
- C++ Pointer to Classes
- C++ Constructors
- C++ Constructor & Destructor
- C++ Default Constructors
- C++ Parameterized Constructors
- C++ Copy Constructor
- C++ Constructor Overloading
- C++ Constructor with Default Arguments
- C++ Delegating Constructors
- C++ Constructor Initialization List
- C++ Dynamic Initialization Using Constructors
- C++ Object-oriented
- C++ Overloading
- C++ Polymorphism
- C++ Abstraction
- C++ Encapsulation
- C++ Interfaces
- C++ Virtual Function
- C++ Pure Virtual Functions & Abstract Classes
- C++ File Handling
- C++ Files and Streams
- C++ Reading From File
- C++ Advanced
- C++ Exception Handling
- C++ Dynamic Memory
- C++ Namespaces
- C++ Templates
- C++ Preprocessor
- C++ Signal Handling
- C++ Multithreading
- C++ Web Programming
- C++ Socket Programming
- C++ Concurrency
- C++ Advanced Concepts
- C++ Lambda Expression
- C++ unordered_multiset
C++ Socket Programming
C++ socket programming is the way to establish communication between two sockets on the network using C++. In this tutorial, we will learn all about socket programming using different types of sockets in C++.
What are Sockets?
Sockets act as the contact points for network data exchange, it's like endpoints for sending and receiving data across a network. They allow applications to communicate with one another using protocols such as TCP (Transmission Control Protocol) and UDP (User Datagram Protocol). They are the backbone of most internet communication as it enables us from web browsing to real-time chatting.
There are two types of sockets:
- Stream Sockets (TCP):
It provides reliable, connection-oriented communication where data is sent in a continuous stream making sure that packets arrive in order and without errors. - Datagram Sockets (UDP):
It provides connectionless communication. Where It transfers data in packets independently, but does not guarantee an order or delivery making it sent in a quick and less reliable way.
Socket Programming in C++
Socket programming in C++ is a powerful approach to creating network applications that allow communication between devices over a network using the socket API. This process involves establishing connections between a client and a server, which enables data exchange through protocols like TCP or UDP.
C++ Server-Side Socket (Listening for Connections)
The following methods are used for handling server-side communication:
1. socket()
The socket() is a system call in network programming that creates a new TCP socket in C++ that is defined inside the <sys/socket.h> header file.
Syntax
int sockfd = socket(AF_INET, SOCK_STREAM, 0);
Where,
- int sockfd declares an integer variable that will store the socket file descriptor.
- AF_INET indicates the socket will use the IPv4 address family.
- SOCK_STREAM specifies that the socket will use TCP (a stream-oriented protocol) and,
- 0 lets the system choose the default protocol for the specified address family and socket type (which is TCP in this case).
2. bind()
The bind() method is associated with a socket, with a specific local address and port number which allows the socket to listen for incoming connection on that address.
Syntax
bind(sockfd, (struct sockaddr*)&address, sizeof(address));
Where,
- sockfd is the file descriptor that represents the socket in your program and is used to perform various socket operations
- (struct sockaddr)&address casts the address structure to a generic pointer type for the bind function.
- sizeof(address) specifies the size of the address structure to inform the system how much data to expect.
3. listen()
The listen() function marks the socket as a passive socket which prepares a socket to accept incoming connection requests (for servers).
Syntax
listen(sockfd, 10);
Where,
- sockfd is the file descriptor that represents the socket in your program and is used to perform various socket operations
- 10 is the backlog parameter, which specifies the maximum number of pending connections that can be queued while the server is busy.
4. accept()
The accept() function accepts a new connection from a client (for servers). It extracts the first connection request on the queue of pending connections and creates a new socket for that connection.
Syntax
int clientSocket = accept(sockfd, (struct sockaddr*)&clientAddress, &clientLen);
Where,
- sockfd: It's the socket's file descriptor where it is used to perform various socket operations.
- (struct sockaddr)&address: This is a type cast that converts the pointer type of clientAddress to a pointer of type struct sockaddr*.
- &clientLen: It is a pointer to a variable that holds the size of the clientAddress.
C++ Client-Side Socket (Connecting to a Server)
The following methods are used for client-side communication:
1. connect()
This function is a system call that attempts to establish a connection to a specified server (for clients) using the socket.
Syntax
connect(sockfd, (struct sockaddr*)&serverAddress, sizeof(serverAddress));
Where,
- sockfd is the file descriptor that represents the socket in your program and is used to perform various socket operations.
- (struct sockaddr*)&serverAddress casts serverAddress to a struct sockaddr* pointer which enables compatibility with functions that require a generic socket address type.
- sizeof(serverAddress) specifies the size of the serverAddress
2. send()
The send() function is a system call in socket programming which sends data to a connected socket.
Syntax
send(sockfd, "Hello", strlen("Hello"), 0);
Where,
- sockfd is the file descriptor that represents the socket in your program and is used to perform various socket operations.
- strlen("Hello") function returns the length of the string "Hello" (5 bytes), showing how many bytes of data to send.
- 0 lets the system choose the default protocol for the specified address family and socket type (which is TCP in this case).
3. recv()
The recv() function is a system call that is used to receive data from a connected socket which allows the client or server to read incoming messages.
Syntax
recv(sockfd, buffer, sizeof(buffer), 0);
Where,
- sockfd is the file descriptor that represents the socket in your program and is used to perform various socket operations.
- buffer is a pointer to the memory location where the received data will be stored. This buffer should be large enough to hold the incoming data.
- sizeof(buffer) specifies the maximum number of bytes to read from the socket, which is typically the size of the buffer.
Closing the Client Socket
The close() method closes an open socket.
Syntax
close(sockfd);
Where,
- close function is a system call that closes the file descriptor associated with the socket.
Required Header Files for Socket Programming
When programming with sockets in C or C++, specific header files must be included for the necessary declarations.
For Linux/Unix Systems
- <sys/socket.h>
- <netinet/in.h>
- <arpa/inet.h>
- <unistd.h>
- <string.h>
- <errno.h>
For Windows Systems
- <winsock2.h>
- <ws2tcpip.h>
- <windows.h>
C++ Example of Socket Programming
Here's a simple Example to illustrate a TCP server and client in C++:
TCP Server Code
#include <netinet/in.h> #include <sys/socket.h> #include <unistd.h> #include <cstring> #include <iostream> #define PORT 8080 int main() { int server_fd, new_socket; struct sockaddr_in address; int opt = 1; int addrlen = sizeof(address); char buffer[1024] = {0}; // Create socket server_fd = socket(AF_INET, SOCK_STREAM, 0); if (server_fd == 0) { perror("socket failed"); exit(EXIT_FAILURE); } // Attach socket to the port setsockopt(server_fd, SOL_SOCKET, SO_REUSEADDR, &opt, sizeof(opt)); address.sin_family = AF_INET; address.sin_addr.s_addr = INADDR_ANY; address.sin_port = htons(PORT); // Bind if (bind(server_fd, (struct sockaddr *)&address, sizeof(address)) < 0) { perror("bind failed"); exit(EXIT_FAILURE); } // Listen if (listen(server_fd, 3) < 0) { perror("listen"); exit(EXIT_FAILURE); } // Accept a connection new_socket = accept(server_fd, (struct sockaddr *)&address, (socklen_t *)&addrlen); if (new_socket < 0) { perror("accept"); exit(EXIT_FAILURE); } // Read data read(new_socket, buffer, 1024); std::cout << "Message from client: " << buffer << std::endl; // Close socket close(new_socket); close(server_fd); return 0; }
TCP Client Code
#include <arpa/inet.h> #include <netinet/in.h> #include <sys/socket.h> #include <unistd.h> #include <cstring> #include <iostream> #define PORT 8080 int main() { int sock = 0; struct sockaddr_in serv_addr; const char *hello = "Hello from client"; // Create socket sock = socket(AF_INET, SOCK_STREAM, 0); if (sock < 0) { std::cerr << "Socket creation error" << std::endl; return -1; } serv_addr.sin_family = AF_INET; serv_addr.sin_port = htons(PORT); // Convert IPv4 and IPv6 addresses from text to binary if (inet_pton(AF_INET, "127.0.0.1", &serv_addr.sin_addr) <= 0) { std::cerr << "Invalid address/ Address not supported" << std::endl; return -1; } // Connect to server if (connect(sock, (struct sockaddr *)&serv_addr, sizeof(serv_addr)) < 0) { std::cerr << "Connection Failed" << std::endl; return -1; } // Send data send(sock, hello, strlen(hello), 0); std::cout << "Message sent" << std::endl; // Close socket close(sock); return 0; }
Steps to Compile and Run
The following are the steps to compiler and run client socket program:
To compiler both server and client code files:
g++ -o server server.cpp g++ -o client client.cpp
To run the Server:
./server
To run the Client (in another terminal):
./client
Best Practices
- Error Handling: Always check the return values of socket functions to handle errors properly.
- Blocking vs Non-blocking: By default, sockets operate in blocking mode. So, consider using non-blocking sockets or multiplexing (like select or poll) for handling multiple connections.
- Cross-platform Issues: This example is for Unix/Linux. For Windows, you’ll need to include <winsock2.h> and initialize Winsock with WSAStartup().
Real-world Applications
There are various applications of sockets in real-life uses and tools etc, here’s mentioned a few of them.
These examples demonstrate how to use sockets for different applications:
- Echo Server: A straightforward server that echoes received messages.
- Chat Application: A multi-threaded server that allows multiple clients to chat.
- FTP Client/Server: A simple implementation for transferring files over a network.
- Web Server: Sockets handle HTTP requests and responses for serving web content.
- Online Multiplayer Games: Sockets enable real-time communication between players and game servers.
- Remote Access Tools: Sockets provide secure connections for remote administration of servers.
- VoIP Applications: Sockets transmit audio and video data in real time for communication.
- Streaming Services: Sockets deliver continuous audio and video content to users.
- IoT Devices: Sockets facilitate communication between smart devices and servers.
- Real-Time Collaboration Tools: Sockets allow instant sharing of edits and messages among users.
- Data Synchronization Services: Sockets manage file uploads and downloads between devices and servers.
- Weather Monitoring Systems: Sockets send real-time weather data to central servers for analysis.
- Payment Processing Systems: Sockets securely transmit transaction data between clients and banks.
- Chatbots: Sockets enable immediate messaging and responses in conversational interfaces.