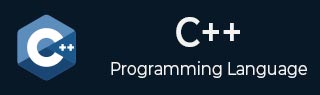
- C++ Home
- C++ Overview
- C++ Environment Setup
- C++ Basic Syntax
- C++ Comments
- C++ Hello World
- C++ Omitting Namespace
- C++ Tokens
- C++ Constants/Literals
- C++ Keywords
- C++ Identifiers
- C++ Data Types
- C++ Numeric Data Types
- C++ Character Data Type
- C++ Boolean Data Type
- C++ Variable Types
- C++ Variable Scope
- C++ Multiple Variables
- C++ Basic Input/Output
- C++ Modifier Types
- C++ Storage Classes
- C++ Numbers
- C++ Enumeration
- C++ Enum Class
- C++ References
- C++ Date & Time
- C++ Operators
- C++ Arithmetic Operators
- C++ Relational Operators
- C++ Logical Operators
- C++ Bitwise Operators
- C++ Assignment Operators
- C++ sizeof Operator
- C++ Conditional Operator
- C++ Comma Operator
- C++ Member Operators
- C++ Casting Operators
- C++ Pointer Operators
- C++ Operators Precedence
- C++ Unary Operators
- C++ Control Statements
- C++ Decision Making
- C++ if Statement
- C++ if else Statement
- C++ Nested if Statements
- C++ switch Statement
- C++ Nested switch Statements
- C++ Loop Types
- C++ while Loop
- C++ for Loop
- C++ do while Loop
- C++ Foreach Loop
- C++ Nested Loops
- C++ break Statement
- C++ continue Statement
- C++ goto Statement
- C++ Strings
- C++ Strings
- C++ Loop Through a String
- C++ String Length
- C++ String Concatenation
- C++ String Comparison
- C++ Functions
- C++ Functions
- C++ Multiple Function Parameters
- C++ Recursive Function
- C++ Return Values
- C++ Function Overloading
- C++ Function Overriding
- C++ Default Arguments
- C++ Arrays
- C++ Arrays
- C++ Multidimensional Arrays
- C++ Pointer to an Array
- C++ Passing Arrays to Functions
- C++ Return Array from Functions
- C++ Structure & Union
- C++ Structures
- C++ Unions
- C++ Pointers
- C++ Pointers
- C++ Dereferencing
- C++ Modify Pointers
- C++ Class and Objects
- C++ Object Oriented
- C++ Classes & Objects
- C++ Class Member Functions
- C++ Class Access Modifiers
- C++ Static Class Members
- C++ Static Data Members
- C++ Static Member Function
- C++ Inline Functions
- C++ this Pointer
- C++ Friend Functions
- C++ Pointer to Classes
- C++ Constructors
- C++ Constructor & Destructor
- C++ Default Constructors
- C++ Parameterized Constructors
- C++ Copy Constructor
- C++ Constructor Overloading
- C++ Constructor with Default Arguments
- C++ Delegating Constructors
- C++ Constructor Initialization List
- C++ Dynamic Initialization Using Constructors
- C++ Object-oriented
- C++ Overloading
- C++ Polymorphism
- C++ Abstraction
- C++ Encapsulation
- C++ Interfaces
- C++ Virtual Function
- C++ Pure Virtual Functions & Abstract Classes
- C++ File Handling
- C++ Files and Streams
- C++ Reading From File
- C++ Advanced
- C++ Exception Handling
- C++ Dynamic Memory
- C++ Namespaces
- C++ Templates
- C++ Preprocessor
- C++ Signal Handling
- C++ Multithreading
- C++ Web Programming
- C++ Socket Programming
- C++ Concurrency
- C++ Advanced Concepts
- C++ Lambda Expression
- C++ unordered_multiset
Dynamic Initialization Using Constructors in C++
Dynamic Initialization Using Constructors
In C++, Dynamic initialization is the process of initializing variables or objects at runtime using constructors.
Where constructors play an important role in object creation and can be used to initialize both static and dynamic data members of a class.
While creating an object, its constructor is called and if the constructor contains logic to initialize the data members with values, is known as dynamic initialization. This is helpful because here the value is calculated, retrieved, or determined during runtime, which is more flexible than static initialization.
Syntax
Here is the following syntax for dynamic initialization using constructors.
ClassName* objectName = new ClassName(constructor_arguments);
Here, ClassName is the class type.
objectName is the pointer to the object.
constructor_arguments are the arguments passed to the constructor.
Example of Dynamic Initialization Using Constructors
Here is the following example of dynamic initialization using constructors.
#include <iostream> using namespace std; class Rectangle { public: int width, height; // Constructor to initialize width and height Rectangle(int w, int h) : width(w), height(h) {} void display() { cout << "Width: " << width << ", Height: " << height << endl; } }; int main() { // Dynamically creating a Rectangle object using the constructor Rectangle* rect = new Rectangle(10, 5); // Display the values rect->display(); // Deallocate memory delete rect; return 0; }
Output
Width: 10, Height: 5
Explanation
- The new Rectangle(10, 5) dynamically created a Rectangle object having width 10 and height 5 using the constructor.
- This rect->display() is displaying the rectangle's dimensions.
- The delete rect; deallocates the memory used by the Rectangle object.
Why Use Constructors for Dynamic Initialization?
- Allows initialization with values known only at runtime.
- Simplifies object creation and initialization logic.
- Combines initialization and validation in a single step.
Using a constructor to initialize dynamically within C++ makes it so much easier to create an object where the values get determined only at runtime. Encapsulation of initialization logic within the constructor makes the code clean, efficient, and more maintainable; use it whenever object initialization depends upon runtime data.