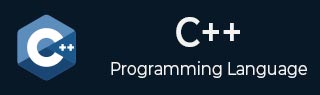
- C++ Home
- C++ Overview
- C++ Environment Setup
- C++ Basic Syntax
- C++ Comments
- C++ Hello World
- C++ Omitting Namespace
- C++ Tokens
- C++ Constants/Literals
- C++ Keywords
- C++ Identifiers
- C++ Data Types
- C++ Numeric Data Types
- C++ Character Data Type
- C++ Boolean Data Type
- C++ Variable Types
- C++ Variable Scope
- C++ Multiple Variables
- C++ Basic Input/Output
- C++ Modifier Types
- C++ Storage Classes
- C++ Numbers
- C++ Enumeration
- C++ Enum Class
- C++ References
- C++ Date & Time
- C++ Operators
- C++ Arithmetic Operators
- C++ Relational Operators
- C++ Logical Operators
- C++ Bitwise Operators
- C++ Assignment Operators
- C++ sizeof Operator
- C++ Conditional Operator
- C++ Comma Operator
- C++ Member Operators
- C++ Casting Operators
- C++ Pointer Operators
- C++ Operators Precedence
- C++ Unary Operators
- C++ Control Statements
- C++ Decision Making
- C++ if Statement
- C++ if else Statement
- C++ Nested if Statements
- C++ switch Statement
- C++ Nested switch Statements
- C++ Loop Types
- C++ while Loop
- C++ for Loop
- C++ do while Loop
- C++ Foreach Loop
- C++ Nested Loops
- C++ break Statement
- C++ continue Statement
- C++ goto Statement
- C++ Strings
- C++ Strings
- C++ Loop Through a String
- C++ String Length
- C++ String Concatenation
- C++ String Comparison
- C++ Functions
- C++ Functions
- C++ Multiple Function Parameters
- C++ Recursive Function
- C++ Return Values
- C++ Function Overloading
- C++ Function Overriding
- C++ Default Arguments
- C++ Arrays
- C++ Arrays
- C++ Multidimensional Arrays
- C++ Pointer to an Array
- C++ Passing Arrays to Functions
- C++ Return Array from Functions
- C++ Structure & Union
- C++ Structures
- C++ Unions
- C++ Pointers
- C++ Pointers
- C++ Dereferencing
- C++ Modify Pointers
- C++ Class and Objects
- C++ Object Oriented
- C++ Classes & Objects
- C++ Class Member Functions
- C++ Class Access Modifiers
- C++ Static Class Members
- C++ Static Data Members
- C++ Static Member Function
- C++ Inline Functions
- C++ this Pointer
- C++ Friend Functions
- C++ Pointer to Classes
- C++ Constructors
- C++ Constructor & Destructor
- C++ Default Constructors
- C++ Parameterized Constructors
- C++ Copy Constructor
- C++ Constructor Overloading
- C++ Constructor with Default Arguments
- C++ Delegating Constructors
- C++ Constructor Initialization List
- C++ Dynamic Initialization Using Constructors
- C++ Object-oriented
- C++ Overloading
- C++ Polymorphism
- C++ Abstraction
- C++ Encapsulation
- C++ Interfaces
- C++ Virtual Function
- C++ Pure Virtual Functions & Abstract Classes
- C++ File Handling
- C++ Files and Streams
- C++ Reading From File
- C++ Advanced
- C++ Exception Handling
- C++ Dynamic Memory
- C++ Namespaces
- C++ Templates
- C++ Preprocessor
- C++ Signal Handling
- C++ Multithreading
- C++ Web Programming
- C++ Socket Programming
- C++ Concurrency
- C++ Advanced Concepts
- C++ Lambda Expression
- C++ unordered_multiset
C++ goto Statement
The goto Statement
A goto statement provides an unconditional jump from the goto to a labeled statement in the same function.
NOTE − Use of goto statement is highly discouraged because it makes difficult to trace the control flow of a program, making the program hard to understand and hard to modify. Any program that uses a goto can be rewritten so that it doesn't need the goto.
The goto statement in C++ is a kind of control flow construct that lets the program jump directly to a given statement within the same function. The goto statement transfers control to a given label in the function. A label is defined with an identifier followed by a colon (:).
Syntax
The syntax of a goto statement in C++ is given as follow −
goto label; .. . label: statement;
Where, label is an identifier that identifies a labeled statement.
A labeled statement is any statement that is preceded by an identifier followed by a colon (:).
Flow Diagram
Here is the following flow diagram, showcasing the working of goto statement-
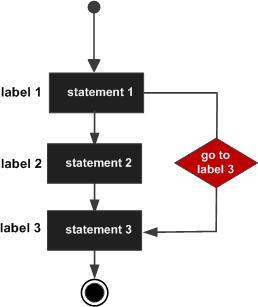
Example
Here is the following example code:
#include <iostream> using namespace std; int main () { // Local variable declaration: int a = 10; // do loop execution LOOP:do { if( a == 15) { // skip the iteration. a = a + 1; goto LOOP; } cout << "value of a: " << a << endl; a = a + 1; } while( a < 20 ); return 0; }
Output
When the above code is compiled and executed, it produces the following result −
value of a: 10 value of a: 11 value of a: 12 value of a: 13 value of a: 14 value of a: 16 value of a: 17 value of a: 18 value of a: 19
One good use of goto is to exit from a deeply nested routine. For example, consider the following code fragment −
for(...) { for(...) { while(...) { if(...) goto stop; . . . } } } stop: cout << "Error in program.\n";
Eliminating the goto would force a number of additional tests to be performed. A simple break statement would not work here, because it would only cause the program to exit from the innermost loop.
When to Use goto?
The goto statement generally is considered unsatisfactory to modern programming style because it causes code to become unstructured. However, it might be useful in the following cases:
- Breaking out of deeply nested loops.
- Handling errors or exceptional cases in legacy code.
Example: Breaking Out of Nested Loops
#include <iostream> using namespace std; int main() { for (int i = 0; i < 3; ++i) { for (int j = 0; j < 3; ++j) { if (i == 1 && j == 1) { goto exitLoops; } cout << "i: " << i << ", j: " << j << endl; } } exitLoops: cout << "Exited the nested loops!" << endl; return 0; }
Output
i: 0, j: 0 i: 0, j: 1 i: 0, j: 2 i: 1, j: 0 Exited the nested loops!