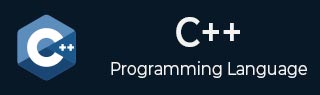
- C++ Home
- C++ Overview
- C++ Environment Setup
- C++ Basic Syntax
- C++ Comments
- C++ Hello World
- C++ Omitting Namespace
- C++ Tokens
- C++ Constants/Literals
- C++ Keywords
- C++ Identifiers
- C++ Data Types
- C++ Numeric Data Types
- C++ Character Data Type
- C++ Boolean Data Type
- C++ Variable Types
- C++ Variable Scope
- C++ Multiple Variables
- C++ Basic Input/Output
- C++ Modifier Types
- C++ Storage Classes
- C++ Numbers
- C++ Enumeration
- C++ Enum Class
- C++ References
- C++ Date & Time
- C++ Operators
- C++ Arithmetic Operators
- C++ Relational Operators
- C++ Logical Operators
- C++ Bitwise Operators
- C++ Assignment Operators
- C++ sizeof Operator
- C++ Conditional Operator
- C++ Comma Operator
- C++ Member Operators
- C++ Casting Operators
- C++ Pointer Operators
- C++ Operators Precedence
- C++ Unary Operators
- C++ Control Statements
- C++ Decision Making
- C++ if Statement
- C++ if else Statement
- C++ Nested if Statements
- C++ switch Statement
- C++ Nested switch Statements
- C++ Loop Types
- C++ while Loop
- C++ for Loop
- C++ do while Loop
- C++ Foreach Loop
- C++ Nested Loops
- C++ break Statement
- C++ continue Statement
- C++ goto Statement
- C++ Strings
- C++ Strings
- C++ Loop Through a String
- C++ String Length
- C++ String Concatenation
- C++ String Comparison
- C++ Functions
- C++ Functions
- C++ Multiple Function Parameters
- C++ Recursive Function
- C++ Return Values
- C++ Function Overloading
- C++ Function Overriding
- C++ Default Arguments
- C++ Arrays
- C++ Arrays
- C++ Multidimensional Arrays
- C++ Pointer to an Array
- C++ Passing Arrays to Functions
- C++ Return Array from Functions
- C++ Structure & Union
- C++ Structures
- C++ Unions
- C++ Pointers
- C++ Pointers
- C++ Dereferencing
- C++ Modify Pointers
- C++ Class and Objects
- C++ Object Oriented
- C++ Classes & Objects
- C++ Class Member Functions
- C++ Class Access Modifiers
- C++ Static Class Members
- C++ Static Data Members
- C++ Static Member Function
- C++ Inline Functions
- C++ this Pointer
- C++ Friend Functions
- C++ Pointer to Classes
- C++ Constructors
- C++ Constructor & Destructor
- C++ Default Constructors
- C++ Parameterized Constructors
- C++ Copy Constructor
- C++ Constructor Overloading
- C++ Constructor with Default Arguments
- C++ Delegating Constructors
- C++ Constructor Initialization List
- C++ Dynamic Initialization Using Constructors
- C++ Object-oriented
- C++ Overloading
- C++ Polymorphism
- C++ Abstraction
- C++ Encapsulation
- C++ Interfaces
- C++ Virtual Function
- C++ Pure Virtual Functions & Abstract Classes
- C++ File Handling
- C++ Files and Streams
- C++ Reading From File
- C++ Advanced
- C++ Exception Handling
- C++ Dynamic Memory
- C++ Namespaces
- C++ Templates
- C++ Preprocessor
- C++ Signal Handling
- C++ Multithreading
- C++ Web Programming
- C++ Socket Programming
- C++ Concurrency
- C++ Advanced Concepts
- C++ Lambda Expression
- C++ unordered_multiset
C++ Logical Operators
Logical operators perform logical operations on Boolean values or expressions. These operators are used to combine two or more conditions and help in decision-making.
Types of Logical Operators
C++ provides three logical operators:
- Logical AND (&&)
- Logical OR (||)
- Logical NOT (!)
1. Logical AND (&&)
The logical AND operator returns true only if both operands are true. If any operand is false, the result is false.
The syntax of logical AND is:
condition_1 && condition 2
2. Logical OR (||)
The logical OR operator returns true if at least one of the conditions is true. If both are false, it returns false.
The syntax of logical OR is:
condition_1 || condition 2
3. Logical NOT (!)
The logical NOT operator negates the given condition. If a condition is true, it returns false and vice versa.
The syntax of logical NOT is:
!condition
Example of Logical Operators
Try the following example to understand all the logical operators available in C++.
Copy and paste the following C++ program in test.cpp file and compile and run this program.
#include <iostream> using namespace std; main() { int a = 5; int b = 20; int c ; if(a && b) { cout << "Line 1 - Condition is true"<< endl ; } if(a || b) { cout << "Line 2 - Condition is true"<< endl ; } /* Let's change the values of a and b */ a = 0; b = 10; if(a && b) { cout << "Line 3 - Condition is true"<< endl ; } else { cout << "Line 4 - Condition is not true"<< endl ; } if(!(a && b)) { cout << "Line 5 - Condition is true"<< endl ; } return 0; }
When the above code is compiled and executed, it produces the following result −
Line 1 - Condition is true Line 2 - Condition is true Line 4 - Condition is not true Line 5 - Condition is true