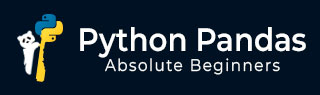
- Python Pandas - Home
- Python Pandas - Introduction
- Python Pandas - Environment Setup
- Python Pandas - Basics
- Python Pandas - Introduction to Data Structures
- Python Pandas - Index Objects
- Python Pandas - Panel
- Python Pandas - Basic Functionality
- Python Pandas - Indexing & Selecting Data
- Python Pandas - Series
- Python Pandas - Series
- Python Pandas - Slicing a Series Object
- Python Pandas - Attributes of a Series Object
- Python Pandas - Arithmetic Operations on Series Object
- Python Pandas - Converting Series to Other Objects
- Python Pandas - DataFrame
- Python Pandas - DataFrame
- Python Pandas - Accessing DataFrame
- Python Pandas - Slicing a DataFrame Object
- Python Pandas - Modifying DataFrame
- Python Pandas - Removing Rows from a DataFrame
- Python Pandas - Arithmetic Operations on DataFrame
- Python Pandas - IO Tools
- Python Pandas - IO Tools
- Python Pandas - Working with CSV Format
- Python Pandas - Reading & Writing JSON Files
- Python Pandas - Reading Data from an Excel File
- Python Pandas - Writing Data to Excel Files
- Python Pandas - Working with HTML Data
- Python Pandas - Clipboard
- Python Pandas - Working with HDF5 Format
- Python Pandas - Comparison with SQL
- Python Pandas - Data Handling
- Python Pandas - Sorting
- Python Pandas - Reindexing
- Python Pandas - Iteration
- Python Pandas - Concatenation
- Python Pandas - Statistical Functions
- Python Pandas - Descriptive Statistics
- Python Pandas - Working with Text Data
- Python Pandas - Function Application
- Python Pandas - Options & Customization
- Python Pandas - Window Functions
- Python Pandas - Aggregations
- Python Pandas - Merging/Joining
- Python Pandas - MultiIndex
- Python Pandas - Basics of MultiIndex
- Python Pandas - Indexing with MultiIndex
- Python Pandas - Advanced Reindexing with MultiIndex
- Python Pandas - Renaming MultiIndex Labels
- Python Pandas - Sorting a MultiIndex
- Python Pandas - Binary Operations
- Python Pandas - Binary Comparison Operations
- Python Pandas - Boolean Indexing
- Python Pandas - Boolean Masking
- Python Pandas - Data Reshaping & Pivoting
- Python Pandas - Pivoting
- Python Pandas - Stacking & Unstacking
- Python Pandas - Melting
- Python Pandas - Computing Dummy Variables
- Python Pandas - Categorical Data
- Python Pandas - Categorical Data
- Python Pandas - Ordering & Sorting Categorical Data
- Python Pandas - Comparing Categorical Data
- Python Pandas - Handling Missing Data
- Python Pandas - Missing Data
- Python Pandas - Filling Missing Data
- Python Pandas - Interpolation of Missing Values
- Python Pandas - Dropping Missing Data
- Python Pandas - Calculations with Missing Data
- Python Pandas - Handling Duplicates
- Python Pandas - Duplicated Data
- Python Pandas - Counting & Retrieving Unique Elements
- Python Pandas - Duplicated Labels
- Python Pandas - Grouping & Aggregation
- Python Pandas - GroupBy
- Python Pandas - Time-series Data
- Python Pandas - Date Functionality
- Python Pandas - Timedelta
- Python Pandas - Sparse Data Structures
- Python Pandas - Sparse Data
- Python Pandas - Visualization
- Python Pandas - Visualization
- Python Pandas - Additional Concepts
- Python Pandas - Caveats & Gotchas
Python Pandas - Time Span Representation
Pandas provides the Period object to represent time spans, also known as regular intervals of time, such as days, months, or years. This is particularly useful when working with time series data that focuses on time periods rather than exact timestamps.
In this tutorial, we will learn about the basics of working with Period objects in Pandas, including how to create and manipulate them, perform arithmetic operations, convert them to Timestamp objects, and obtain formatted string representations.
The Period Object
The Period object in Pandas represents a fixed time span (a period of time), such as a month, a quarter, or a day. You can define the span of a period using the freq parameter, which is set with a frequency alias like "D" for days, "M" for months, or "Y" for years.
Creating a Period object in Pandas can be done by using the pandas.Period() class.
Syntax
Following is the syntax of the Period class−
class pandas.Period(value=None, freq=None, ordinal=None, year=None, month=None, quarter=None, day=None, hour=None, minute=None, second=None)
Example: Creating the Period object
Here is an example of creating the Period object for different frequencies using the pandas.Period() class.
import pandas as pd # Creating Period objects with different frequencies # Yearly frequency p1 = pd.Period("2024", freq="Y") # Daily frequency p2 = pd.Period("2024-01-01", freq="D") # Hourly frequency p3 = pd.Period("2024-01-01 19:00", freq="H") print("Output Yearly frequency:", p1) print("Output Daily frequency:", p2) print("Output Hourly frequency:", p3)
On executing the above code you will get the following output −
Output Yearly frequency: 2024 Output Daily frequency: 2024-01-01 Output Hourly frequency: 2024-01-01 19:00
Arithmetic with Period Objects
Pandas Period object support arithmetic operations, like addition and subtraction, based on their defined frequency. You can add or subtract integers from a Period to shift it to that own frequency. Note that arithmetic operations between different frequencies are not allowed.
Example
The following example demonstrates applying the arithmetic operations to Period object with integers.
import pandas as pd # Creating Period objects with different frequencies # Yearly frequency p1 = pd.Period("2024", freq="Y") # Daily frequency p2 = pd.Period("2024-01-01", freq="D") # Hourly frequency p3 = pd.Period("2024-01-01 19:00", freq="H") # Adding and subtracting intervals print("2024+1 =", p1 + 1) print("2024 - 3 =", p1 - 3) print("2024-01-01 + 2 =", p2 + 2) print("2024-01-01 - 2 =", p2 - 2) print("2024-01-01 19:00 + 4 =", p3 + 4) print("2024-01-01 19:00 - 3 =", p3 - 3)
Following is the output of the above code −
2024+1 = 2025 2024 - 3 = 2021 2024-01-01 + 2 = 2024-01-03 2024-01-01 - 2 = 2023-12-30 2024-01-01 19:00 + 4 = 2024-01-01 23:00 2024-01-01 19:00 - 3 = 2024-01-01 16:00
Combining Periods with Time Offsets
If a Period frequency is daily or higher (e.g., hourly, minute, seconds, etc.), you can add time offsets, such as pd.offsets.Hour(2) or datetime.timedelta objects to the Period.
Example
This example demonstrates combining time offsets with a Period object.
import pandas as pd from datetime import timedelta import numpy as np # Create a period object p = pd.Period("2024-11-01 09:00", freq="H") # Adding hours using different timedelta like objects print(p + pd.offsets.Hour(2)) print(p + timedelta(minutes=120)) print(p + np.timedelta64(7200, "s"))
Following is the output of the above code −
2024-11-01 11:00 2024-11-01 11:00 2024-11-01 11:00
Note: you will get the IncompatibleFrequency error, if you try to add a time offset which results can have the different frequency.
Converting a Period Object to a String
The pandas.Period() class provides the strftime() method to convert a period object to a string representation, with custom formatting based on date and time directives.
Example
This example demonstrates the process of formatting and retrieving the string representation of the Period object using the Period.strftime() method.
import pandas as pd period = pd.Period(freq="S", year=2024, month=11, day=5, hour=8, minute=20, second=45) print("Period:", period) # Period object to String representation with specific format print("Formatted String:", period.strftime('%d-%b-%Y')) # Using multiple formatting directives print("Detailed Format:", period.strftime('%b. %d, %Y was a %A'))
Following is the output of the above code −
Period: 2024-11-05 08:20:45 Formatted String: 05-Nov-2024 Detailed Format: Nov. 05, 2024 was a Tuesday
Convert Period Object to Timestamp
The pandas.Period() class provides the to_timestamp() method to convert period object to timestamp with the specified frequencies.
Example: Basic Period Object Conversion to Timestamp
Here is the basic example of using the pandas.Period.to_timestamp() method to convert period object to timestamp object.
import pandas as pd period = pd.Period(freq="S", year=2024, month=11, day=5, hour=8, minute=20, second=45) print("Period:", period) # Convert to Timestamp print("Converted to Timestamp:", period.to_timestamp(freq='T'))
Following is the output of the above code −
Period: 2024-11-05 08:20:45 Converted to Timestamp: 2024-11-05 08:20:00
Example: Period to Timestamp Conversion with Daily Frequency
This example shows how to convert a Period object to a Timestamp with Daily Frequency.
import pandas as pd period = pd.Period(freq="S", year=2024, month=11, day=5, hour=8, minute=20, second=45) print("Period:", period) # Convert to daily frequency Timestamp print("Daily Timestamp:", period.to_timestamp(freq='D'))
Following is the output of the above code −
Period: 2024-11-05 08:20:45 Daily Timestamp: 2024-11-05 00:00:00
Example: Conversion with Minutely Frequency
Here is another example of converting the Period object to Timestamp with minutely frequency.
import pandas as pd period = pd.Period(freq="S", year=2024, month=11, day=5, hour=8, minute=20, second=45) print("Period:", period) # Convert to minutely frequency Timestamp print("Minutely Timestamp:", period.to_timestamp(freq='T'))
Following is the output of the above code −
Period: 2024-11-05 08:20:45 Minutely Timestamp: 2024-11-05 08:20:00