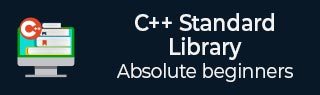
- C++ Library - Home
- C++ Library - <fstream>
- C++ Library - <iomanip>
- C++ Library - <ios>
- C++ Library - <iosfwd>
- C++ Library - <iostream>
- C++ Library - <istream>
- C++ Library - <ostream>
- C++ Library - <sstream>
- C++ Library - <streambuf>
- C++ Library - <atomic>
- C++ Library - <complex>
- C++ Library - <exception>
- C++ Library - <functional>
- C++ Library - <limits>
- C++ Library - <locale>
- C++ Library - <memory>
- C++ Library - <new>
- C++ Library - <numeric>
- C++ Library - <regex>
- C++ Library - <stdexcept>
- C++ Library - <string>
- C++ Library - <thread>
- C++ Library - <tuple>
- C++ Library - <typeinfo>
- C++ Library - <utility>
- C++ Library - <valarray>
- The C++ STL Library
- C++ Library - <array>
- C++ Library - <bitset>
- C++ Library - <deque>
- C++ Library - <forward_list>
- C++ Library - <list>
- C++ Library - <map>
- C++ Library - <multimap>
- C++ Library - <queue>
- C++ Library - <priority_queue>
- C++ Library - <set>
- C++ Library - <stack>
- C++ Library - <unordered_map>
- C++ Library - <unordered_set>
- C++ Library - <vector>
- C++ Library - <algorithm>
- C++ Library - <iterator>
- The C++ Advanced Library
- C++ Library - <any>
- C++ Library - <barrier>
- C++ Library - <bit>
- C++ Library - <chrono>
- C++ Library - <cinttypes>
- C++ Library - <clocale>
- C++ Library - <condition_variable>
- C++ Library - <coroutine>
- C++ Library - <cstdlib>
- C++ Library - <cstring>
- C++ Library - <cuchar>
- C++ Library - <charconv>
- C++ Library - <cfenv>
- C++ Library - <cmath>
- C++ Library - <ccomplex>
- C++ Library - <expected>
- C++ Library - <format>
- C++ Library - <future>
- C++ Library - <flat_set>
- C++ Library - <flat_map>
- C++ Library - <filesystem>
- C++ Library - <generator>
- C++ Library - <initializer_list>
- C++ Library - <latch>
- C++ Library - <memory_resource>
- C++ Library - <mutex>
- C++ Library - <mdspan>
- C++ Library - <optional>
- C++ Library - <print>
- C++ Library - <ratio>
- C++ Library - <scoped_allocator>
- C++ Library - <semaphore>
- C++ Library - <source_location>
- C++ Library - <span>
- C++ Library - <spanstream>
- C++ Library - <stacktrace>
- C++ Library - <stop_token>
- C++ Library - <syncstream>
- C++ Library - <system_error>
- C++ Library - <string_view>
- C++ Library - <stdatomic>
- C++ Library - <variant>
- C++ STL Library Cheat Sheet
- C++ STL - Cheat Sheet
- C++ Programming Resources
- C++ Programming Tutorial
- C++ Useful Resources
- C++ Discussion
C++ Library - <syncstream>
The <syncstream> header in C++20, provides a convenient way to manage output streams in a thread safe manner. With the rise of multithreading in modern applications, ensuring that output from different threads does not interfere with each other has become important. Traditional output streams (like std::cout) are not thread-safe, which lead to data races and unpredictable output when multiple threads attempt to write the same stream simultaneously.
The <syncstream> header addresses this by introducing the std::syncbuf class, which allows to create a synchronized output streams. These streams ensure that output operations are atomic, meaning that when one thread is writing to a synchronized stream, other threads are blocked from writing untill the operation is complete.
Including <syncstream> Header
To include the <syncstream> header in your C++ program, you can use the following syntax.
#include <syncstream>
Functions of <span> Header
Below is list of all functions from <span> header.
Sr.No | Functions & Description |
---|---|
1 | operator= It assigns a basic_syncbuf object. |
2 | swap It swaps two basic_syncbuf objects. |
3 | emit It atomically transmits the entire internal buffer to the wrapped streambuf. |
4 | get_wrapped It retrieves the wrapped streambuf pointer. |
5 | get_allocator It retrieves the allocator used by this basic_syncbuf. |
6 | set_emit_on_sync It changes the current emit-on-sync policy. |
7 | sync It either emits, or records a pending flush, depending on the current emit-on-sync policy. |
8 | rdbuf It obtains a pointer to the underlying basic_syncbuf. |
Flushing Multiple Outputs Together
In the following example, we are going to combine the multiple output messages into a single flush.
#include <iostream> #include <syncstream> int main() { std::osyncstream a(std::cout); a << "Hi, " << "Hello, " << "Welcome" << std::endl; return 0; }
Output
Following is the output of the above code −
Hi, Hello, Welcome
Manual Control of Flushing
Consider the following example, where we are going to manually flush the output using the flush() function.
#include <iostream> #include <syncstream> #include <chrono> #include <thread> int main() { std::osyncstream a(std::cout); a << "Welcome."; a.flush(); std::this_thread::sleep_for(std::chrono::seconds(3)); a << "Visit Again!" << std::endl; return 0; }
Output
Output of the above code is as follows −
Welcome.Visit Again!