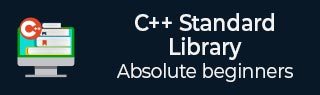
- C++ Library - Home
- C++ Library - <fstream>
- C++ Library - <iomanip>
- C++ Library - <ios>
- C++ Library - <iosfwd>
- C++ Library - <iostream>
- C++ Library - <istream>
- C++ Library - <ostream>
- C++ Library - <sstream>
- C++ Library - <streambuf>
- C++ Library - <atomic>
- C++ Library - <complex>
- C++ Library - <exception>
- C++ Library - <functional>
- C++ Library - <limits>
- C++ Library - <locale>
- C++ Library - <memory>
- C++ Library - <new>
- C++ Library - <numeric>
- C++ Library - <regex>
- C++ Library - <stdexcept>
- C++ Library - <string>
- C++ Library - <thread>
- C++ Library - <tuple>
- C++ Library - <typeinfo>
- C++ Library - <utility>
- C++ Library - <valarray>
- The C++ STL Library
- C++ Library - <array>
- C++ Library - <bitset>
- C++ Library - <deque>
- C++ Library - <forward_list>
- C++ Library - <list>
- C++ Library - <map>
- C++ Library - <multimap>
- C++ Library - <queue>
- C++ Library - <priority_queue>
- C++ Library - <set>
- C++ Library - <stack>
- C++ Library - <unordered_map>
- C++ Library - <unordered_set>
- C++ Library - <vector>
- C++ Library - <algorithm>
- C++ Library - <iterator>
- The C++ Advanced Library
- C++ Library - <any>
- C++ Library - <barrier>
- C++ Library - <bit>
- C++ Library - <chrono>
- C++ Library - <cinttypes>
- C++ Library - <clocale>
- C++ Library - <condition_variable>
- C++ Library - <coroutine>
- C++ Library - <cstdlib>
- C++ Library - <cstring>
- C++ Library - <cuchar>
- C++ Library - <charconv>
- C++ Library - <cfenv>
- C++ Library - <cmath>
- C++ Library - <ccomplex>
- C++ Library - <expected>
- C++ Library - <format>
- C++ Library - <future>
- C++ Library - <flat_set>
- C++ Library - <flat_map>
- C++ Library - <filesystem>
- C++ Library - <generator>
- C++ Library - <initializer_list>
- C++ Library - <latch>
- C++ Library - <memory_resource>
- C++ Library - <mutex>
- C++ Library - <mdspan>
- C++ Library - <optional>
- C++ Library - <print>
- C++ Library - <ratio>
- C++ Library - <scoped_allocator>
- C++ Library - <semaphore>
- C++ Library - <source_location>
- C++ Library - <span>
- C++ Library - <spanstream>
- C++ Library - <stacktrace>
- C++ Library - <stop_token>
- C++ Library - <syncstream>
- C++ Library - <system_error>
- C++ Library - <string_view>
- C++ Library - <stdatomic>
- C++ Library - <variant>
- C++ STL Library Cheat Sheet
- C++ STL - Cheat Sheet
- C++ Programming Resources
- C++ Programming Tutorial
- C++ Useful Resources
- C++ Discussion
C++ Library - <ratio>
The <ratio> library in C++ provides a convenient way to work with the rational numbers at compile time. It is used for defining and manipulating fractional values in terms of numerator and denominator. It is used in the scenarios where the fractional arithmetic needs to be calculated and optimized during the compilation phase, ensuring that there is no runtime overhead.
The <ratio> library is widely used in combination with other libraries such as <chrono> for handling time units. The commonly used C++ <ratio> functions are listed below.
Including <ratio> Header
To include the <ratio> header in your C++ program, you can use the following syntax.
#include <ratio>
Functions of <ratio> Header
Below is list of all functions from <ratio> header.
Arithmetic
The arithmetic refers to the ability to perform basic mathematical operations on compile-time. The commonly used C++ <ratio> arithmetic functions are listed below along with their description.
Sr.No | Function & Description |
---|---|
1 | ratio_add It is used to add two ratio objects. |
2 | ratio_subtract It is used to subtract two ratio objects. |
3 | ratio_multiply It is used to multiply two ratio objects. |
4 | ratio_divide It is used to divide two ratio objects. |
Adding Two Ratios
In the following example, we are going to declare the two ratios and retrieving the output as the sum of the both ratios.
#include <iostream> #include <ratio> int main() { using x1 = std::ratio < 2, 4 > ; using x2 = std::ratio < 1, 4 > ; using a = std::ratio_add < x1, x2 > ; std::cout << "Result : " << a::num << "/" << a::den << std::endl; return 0; }
Output
Following is the output of the above code −
Result : 3/4
Comparison
The <ratio> library provides a way ro compare different std::ratio types at compile-time using the following comparison functions that are listed below along with their description.
Sr.No | Function & Description |
---|---|
1 | ratio_equal It is used to compare two ratio objects for equality. |
2 | ratio_not_equal It is used to compare two ratio objects for inequality. |
3 | ratio_less It is used to compare two ratio objects for less than. |
4 | ratio_less_equal It is used to compare two ratio objects for less than or equal. |
5 | ratio_greater It is used to compare two ratio objects for greater than. |
6 | ratio_greater_equal It is used to compare two ratio objects for greater than or equal. |
Comparing Two Ratios
Consider the following example, where we are going to declare two ratios and comparing them.
#include <iostream> #include <ratio> int main() { using a1 = std::ratio < 1, 3 > ; using a2 = std::ratio < 3, 9 > ; if (std::ratio_equal < a1, a2 > ::value) { std::cout << "Both Ratios are Equal" << std::endl; } else { std::cout << "Both Ratios are Not Equal" << std::endl; } return 0; }
Output
Output of the above code is as follows −
Both Ratios are Equal