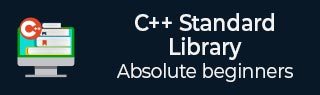
- C++ Library - Home
- C++ Library - <fstream>
- C++ Library - <iomanip>
- C++ Library - <ios>
- C++ Library - <iosfwd>
- C++ Library - <iostream>
- C++ Library - <istream>
- C++ Library - <ostream>
- C++ Library - <sstream>
- C++ Library - <streambuf>
- C++ Library - <atomic>
- C++ Library - <complex>
- C++ Library - <exception>
- C++ Library - <functional>
- C++ Library - <limits>
- C++ Library - <locale>
- C++ Library - <memory>
- C++ Library - <new>
- C++ Library - <numeric>
- C++ Library - <regex>
- C++ Library - <stdexcept>
- C++ Library - <string>
- C++ Library - <thread>
- C++ Library - <tuple>
- C++ Library - <typeinfo>
- C++ Library - <utility>
- C++ Library - <valarray>
- The C++ STL Library
- C++ Library - <array>
- C++ Library - <bitset>
- C++ Library - <deque>
- C++ Library - <forward_list>
- C++ Library - <list>
- C++ Library - <map>
- C++ Library - <multimap>
- C++ Library - <queue>
- C++ Library - <priority_queue>
- C++ Library - <set>
- C++ Library - <stack>
- C++ Library - <unordered_map>
- C++ Library - <unordered_set>
- C++ Library - <vector>
- C++ Library - <algorithm>
- C++ Library - <iterator>
- The C++ Advanced Library
- C++ Library - <any>
- C++ Library - <barrier>
- C++ Library - <bit>
- C++ Library - <chrono>
- C++ Library - <cinttypes>
- C++ Library - <clocale>
- C++ Library - <condition_variable>
- C++ Library - <coroutine>
- C++ Library - <cstdlib>
- C++ Library - <cstring>
- C++ Library - <cuchar>
- C++ Library - <charconv>
- C++ Library - <cfenv>
- C++ Library - <cmath>
- C++ Library - <ccomplex>
- C++ Library - <expected>
- C++ Library - <format>
- C++ Library - <future>
- C++ Library - <flat_set>
- C++ Library - <flat_map>
- C++ Library - <filesystem>
- C++ Library - <generator>
- C++ Library - <initializer_list>
- C++ Library - <latch>
- C++ Library - <memory_resource>
- C++ Library - <mutex>
- C++ Library - <mdspan>
- C++ Library - <optional>
- C++ Library - <print>
- C++ Library - <ratio>
- C++ Library - <scoped_allocator>
- C++ Library - <semaphore>
- C++ Library - <source_location>
- C++ Library - <span>
- C++ Library - <spanstream>
- C++ Library - <stacktrace>
- C++ Library - <stop_token>
- C++ Library - <syncstream>
- C++ Library - <system_error>
- C++ Library - <string_view>
- C++ Library - <stdatomic>
- C++ Library - <variant>
- C++ STL Library Cheat Sheet
- C++ STL - Cheat Sheet
- C++ Programming Resources
- C++ Programming Tutorial
- C++ Useful Resources
- C++ Discussion
C++ Fstream Library - Open Function
Description
Opens the file identified by argument filename, associating it with the stream object, so that input/output operations are performed on its content. Argument mode specifies the opening mode.
Declaration
Following is the declaration for fstream::open.
C++98
void open (const char* filename,ios_base::openmode mode = ios_base::in | ios_base::out);
C++11
void open (const char* filename,ios_base::openmode mode = ios_base::in | ios_base::out); void open (const string& filename,ios_base::openmode mode = ios_base::in | ios_base::out);
Parameters
filename − String with the name of the file to open,Specifics about its format and validity depend on the library implementation and running environment.
mode − Flags describing the requested input/output mode for the file.
Return Value
none
If the function fails to open a file, the failbit state flag is set for the stream (which may throw ios_base::failure if that state flag was registered using member exceptions).
Exceptions
Basic guarantee − if an exception is thrown, the stream is in a valid state.
It throws an exception of member type failure if the function fails (setting the failbit state flag) and member exceptions was set to throw for that state.
Data races
Modifies the fstream object.
Concurrent access to the same stream object introduce data races.
Example
In below example explains about fstream open function.
#include <fstream> int main () { std::fstream fs; fs.open ("test.txt", std::fstream::in | std::fstream::out | std::fstream::app); fs << " more lorem ipsum"; fs.close(); return 0; }