
- TypeScript - Home
- TypeScript - Roadmap
- TypeScript - Overview
- TypeScript - Environment Setup
- TypeScript - Basic Syntax
- TypeScript vs. JavaScript
- TypeScript - Features
- TypeScript - Variables
- TypeScript - let & const
- TypeScript - Operators
- TypeScript - Types
- TypeScript - Type Annotations
- TypeScript - Type Inference
- TypeScript - Numbers
- TypeScript - Strings
- TypeScript - Boolean
- TypeScript - Arrays
- TypeScript - Tuples
- TypeScript - Enums
- TypeScript - Any
- TypeScript - Never
- TypeScript - Union
- TypeScript - Literal Types
- TypeScript - Symbols
- TypeScript - null vs. undefined
- TypeScript - Type Aliases
- TypeScript Control Flow
- TypeScript - Decision Making
- TypeScript - If Statement
- TypeScript - If Else Statement
- TypeScript - Nested If Statements
- TypeScript - Switch Statement
- TypeScript - Loops
- TypeScript - For Loop
- TypeScript - While Loop
- TypeScript - Do While Loop
- TypeScript Functions
- TypeScript - Functions
- TypeScript - Function Types
- TypeScript - Optional Parameters
- TypeScript - Default Parameters
- TypeScript - Anonymous Functions
- TypeScript - Function Constructor
- TypeScript - Rest Parameter
- TypeScript - Parameter Destructuring
- TypeScript - Arrow Functions
- TypeScript Interfaces
- TypeScript - Interfaces
- TypeScript - Extending Interfaces
- TypeScript Classes and Objects
- TypeScript - Classes
- TypeScript - Objects
- TypeScript - Access Modifiers
- TypeScript - Readonly Properties
- TypeScript - Inheritance
- TypeScript - Static Methods and Properties
- TypeScript - Abstract Classes
- TypeScript - Accessors
- TypeScript - Duck-Typing
- TypeScript Advanced Types
- TypeScript - Intersection Types
- TypeScript - Type Guards
- TypeScript - Type Assertions
- TypeScript Type Manipulation
- TypeScript - Creating Types from Types
- TypeScript - Keyof Type Operator
- TypeScript - Typeof Type Operator
- TypeScript - Indexed Access Types
- TypeScript - Conditional Types
- TypeScript - Mapped Types
- TypeScript - Template Literal Types
- TypeScript Generics
- TypeScript - Generics
- TypeScript - Generic Constraints
- TypeScript - Generic Interfaces
- TypeScript - Generic Classes
- TypeScript Miscellaneous
- TypeScript - Triple-Slash Directives
- TypeScript - Namespaces
- TypeScript - Modules
- TypeScript - Ambients
- TypeScript - Decorators
- TypeScript - Type Compatibility
- TypeScript - Date Object
- TypeScript - Iterators and Generators
- TypeScript - Mixins
- TypeScript - Utility Types
- TypeScript - Boxing and Unboxing
- TypeScript - tsconfig.json
- From JavaScript To TypeScript
- TypeScript Useful Resources
- TypeScript - Quick Guide
- TypeScript - Cheatsheet
- TypeScript - Useful Resources
- TypeScript - Discussion
TypeScript - For Loop
The for loop
The for loop executes the code block for a specified number of times. It can be used to iterate over a fixed set of values, such as an array.
The TypeScript offers two additional loop variants: for...in and for...of. These variants are particularly useful for iterating through iterables like arrays, strings, and maps.
The for...of loop is generally preferred for iterating over iterables. It directly accesses the elements within the iterables.
The for...in loop iterates the enumerable properties of an object. While it can be used with arrays or strings, it is generally not recommended for these cases.
Syntax
The syntax of the for loop is as below −
for (initial_count_value; termination-condition; step) { //statements }
The loop uses a count variable to keep track of the iterations. The loop initializes the iteration by setting the value of count to its initial value. It executes the code block, each time the value of count satisfies the termination_condtion. The step changes the value of count after every iteration.
Flowchart
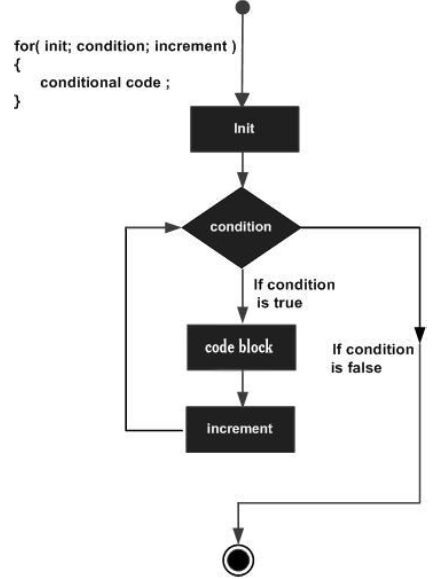
Example: Simple for loop
var num:number = 5; var i:number; var factorial = 1; for(i = num;i>=1;i--) { factorial *= i; } console.log(factorial)
The program calculates the factorial of the number 5 and displays the same. The for loop generates the sequence of numbers from 5 to 1, calculating the product of the numbers in every iteration.
On compiling, it will generate following JavaScript code.
var num = 5; var i; var factorial = 1; for (i = num; i >= 1; i--) { factorial *= i; } console.log(factorial);
The code produces the following output −
120
Example: The for loop with break statement
var i: number = 0; for (i; i < 5; i++) { if (i == 4) { break; } console.log(i); }
Inside the if condition, it breaks and exit the for loop when the value of i equals to 4. So it prints from 0 to 3 only.
On compiling, it will generate the following JavaScript code.
var i = 0; for (i; i < 5; i++) { if (i == 4) { break; } console.log(i); }
The output of the above example is as follows
0 1 2 3
Example: The for loop with continue statement
var i: number = 0; for (i; i < 5; i++) { if (i % 2 == 0) { continue; } console.log(i); }
In the example above, the if condition (i % 2 == 0) evaluates to true, the execution control goes to then next iteration. The statements following the if block are skipped. When condition is false, the statements following the if block get executed.
On compiling it will generate the following JavaScript code.
var i = 0; for (i; i < 5; i++) { if (i % 2 == 0) { continue; } console.log(i); }
The output is as follows
1 3
The for...in loop
Another variation of the for loop is the for... in loop. The for in loop can be used to iterate over a set of values as in the case of an array or a tuple. The syntax for the same is given below −
The for...in loop is used to iterate through a list or collection of values. The data type of val here should be string or any.
Syntax
The syntax of the for..in loop is as given below −
for (var val in list) { //statements }
Lets take a look at the following examples −
Example: The for...in loop with strings
var j:any; var n:any = "abc"; for(j in n) { console.log(n[j]) }
On compiling, it will generate the following JavaScript code
var j; var n = "abc"; for (j in n) { console.log(n[j]); }
It will produce the following output
a b c
Example: The for...in loop with arrays
const arr: number[] = [10,20,30]; for(var idx in arr){ console.log(arr[idx]); }
On compiling it will generate the following JavaScript code.
const arr = [10, 20, 30]; for (var idx in arr) { console.log(arr[idx]); }
The output of the above example code is as follows
10 20 30
Example: The for...in loop with tuples
const tp:[string, number] = ['TypeScript',20] for( var j in tp) { console.log(tp[j]) }
On compiling, it will generate the following JavaScript code.
const tp = ['TypeScript', 20]; for (var j in tp) { console.log(tp[j]); }
The output of the above example code is as follows
TypeScript 20
The for...of loop
The for...of loop in another variant of the for loop. The for...of loop can be used to iterate through the values of iterables. For example, we can use the for...of loop to iterate through the array and get values from every index.
Syntax
The syntax of the for...of loop in TypeScript is as follows
for (var element of iterable){ // statements }
Where,
element − It is a current element of the iterable.
of − It is a ES6 operator in TypeScript.
iterable − It is an iterable like an array, string, etc.
Example: The for...of loop with arrays
const arr: string[] = ["Tutorialspoint", "JavaScript", "TypeScript"]; for (var element of arr) { console.log(element); }
On compiling, it will generate the following JavaScript code.
const arr = ["Tutorialspoint", "JavaScript", "TypeScript"]; for (var element of arr) { console.log(element); }
The output of the above example code is follows
Tutorialspoint JavaScript TypeScript
Example: The for...of loop with strings
let str: string = "Hello"; for (var char of str) { console.log(char); }
On compiling, it will generate the following TypeScript code.
let str = "Hello"; for (var char of str) { console.log(char); }
The output of the above example code is as follows
H e l l o