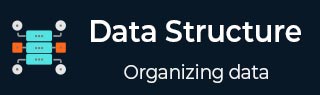
- DSA - Home
- DSA - Overview
- DSA - Environment Setup
- DSA - Algorithms Basics
- DSA - Asymptotic Analysis
- Data Structures
- DSA - Data Structure Basics
- DSA - Data Structures and Types
- DSA - Array Data Structure
- DSA - Skip List Data Structure
- Linked Lists
- DSA - Linked List Data Structure
- DSA - Doubly Linked List Data Structure
- DSA - Circular Linked List Data Structure
- Stack & Queue
- DSA - Stack Data Structure
- DSA - Expression Parsing
- DSA - Queue Data Structure
- DSA - Circular Queue Data Structure
- DSA - Priority Queue Data Structure
- DSA - Deque Data Structure
- Searching Algorithms
- DSA - Searching Algorithms
- DSA - Linear Search Algorithm
- DSA - Binary Search Algorithm
- DSA - Interpolation Search
- DSA - Jump Search Algorithm
- DSA - Exponential Search
- DSA - Fibonacci Search
- DSA - Sublist Search
- DSA - Hash Table
- Sorting Algorithms
- DSA - Sorting Algorithms
- DSA - Bubble Sort Algorithm
- DSA - Insertion Sort Algorithm
- DSA - Selection Sort Algorithm
- DSA - Merge Sort Algorithm
- DSA - Shell Sort Algorithm
- DSA - Heap Sort Algorithm
- DSA - Bucket Sort Algorithm
- DSA - Counting Sort Algorithm
- DSA - Radix Sort Algorithm
- DSA - Quick Sort Algorithm
- Matrices Data Structure
- DSA - Matrices Data Structure
- DSA - Lup Decomposition In Matrices
- DSA - Lu Decomposition In Matrices
- Graph Data Structure
- DSA - Graph Data Structure
- DSA - Depth First Traversal
- DSA - Breadth First Traversal
- DSA - Spanning Tree
- DSA - Topological Sorting
- DSA - Strongly Connected Components
- DSA - Biconnected Components
- DSA - Augmenting Path
- DSA - Network Flow Problems
- DSA - Flow Networks In Data Structures
- DSA - Edmonds Blossom Algorithm
- DSA - Maxflow Mincut Theorem
- Tree Data Structure
- DSA - Tree Data Structure
- DSA - Tree Traversal
- DSA - Binary Search Tree
- DSA - AVL Tree
- DSA - Red Black Trees
- DSA - B Trees
- DSA - B+ Trees
- DSA - Splay Trees
- DSA - Range Queries
- DSA - Segment Trees
- DSA - Fenwick Tree
- DSA - Fusion Tree
- DSA - Hashed Array Tree
- DSA - K-Ary Tree
- DSA - Kd Trees
- DSA - Priority Search Tree Data Structure
- Recursion
- DSA - Recursion Algorithms
- DSA - Tower of Hanoi Using Recursion
- DSA - Fibonacci Series Using Recursion
- Divide and Conquer
- DSA - Divide and Conquer
- DSA - Max-Min Problem
- DSA - Strassen's Matrix Multiplication
- DSA - Karatsuba Algorithm
- Greedy Algorithms
- DSA - Greedy Algorithms
- DSA - Travelling Salesman Problem (Greedy Approach)
- DSA - Prim's Minimal Spanning Tree
- DSA - Kruskal's Minimal Spanning Tree
- DSA - Dijkstra's Shortest Path Algorithm
- DSA - Map Colouring Algorithm
- DSA - Fractional Knapsack Problem
- DSA - Job Sequencing with Deadline
- DSA - Optimal Merge Pattern Algorithm
- Dynamic Programming
- DSA - Dynamic Programming
- DSA - Matrix Chain Multiplication
- DSA - Floyd Warshall Algorithm
- DSA - 0-1 Knapsack Problem
- DSA - Longest Common Sub-sequence Algorithm
- DSA - Travelling Salesman Problem (Dynamic Approach)
- Hashing
- DSA - Hashing Data Structure
- DSA - Collision In Hashing
- Disjoint Set
- DSA - Disjoint Set
- DSA - Path Compression And Union By Rank
- Heap
- DSA - Heap Data Structure
- DSA - Binary Heap
- DSA - Binomial Heap
- DSA - Fibonacci Heap
- Tries Data Structure
- DSA - Tries
- DSA - Standard Tries
- DSA - Compressed Tries
- DSA - Suffix Tries
- Treaps
- DSA - Treaps Data Structure
- Bit Mask
- DSA - Bit Mask In Data Structures
- Bloom Filter
- DSA - Bloom Filter Data Structure
- Approximation Algorithms
- DSA - Approximation Algorithms
- DSA - Vertex Cover Algorithm
- DSA - Set Cover Problem
- DSA - Travelling Salesman Problem (Approximation Approach)
- Randomized Algorithms
- DSA - Randomized Algorithms
- DSA - Randomized Quick Sort Algorithm
- DSA - Karger’s Minimum Cut Algorithm
- DSA - Fisher-Yates Shuffle Algorithm
- Miscellaneous
- DSA - Infix to Postfix
- DSA - Bellmon Ford Shortest Path
- DSA - Maximum Bipartite Matching
- DSA Useful Resources
- DSA - Questions and Answers
- DSA - Selection Sort Interview Questions
- DSA - Merge Sort Interview Questions
- DSA - Insertion Sort Interview Questions
- DSA - Heap Sort Interview Questions
- DSA - Bubble Sort Interview Questions
- DSA - Bucket Sort Interview Questions
- DSA - Radix Sort Interview Questions
- DSA - Cycle Sort Interview Questions
- DSA - Quick Guide
- DSA - Useful Resources
- DSA - Discussion
Bubble Sort Interview Questions and Answers
Bubble sort is the sorting technique that starts by comparing the first two elements, and at the end, it gets the largest element from the first cycle. This cycle runs until the whole unsorted array is sorted. This article covers all the basic level questions to advanced level interview questions. It helps to learn good topics of bubble sort.

Bubble Sort Interview Questions and Answers
Following are the Bubble Sort interview question answers:
1. What is the Bubble Sort?
Bubble Sort is used to compare the two elements from an array in which it starts from the first two elements to the last two elements. It compares only adjacent elements and swaps them if they are in the wrong order. After every cycle, you obtain the highest element of the cycle, and this top element is not taken into consideration in the next cycle. The number of cycles is based on the number of elements in the array.
2. What is the time and space complexity of Bubble Sort?
The worst and best case time complexity of Bubble Sort is O(N), and the average case time complexity is O(N). The space complexity is O(1), as it requires only a constant amount of additional space.
3. What is the nature of the Bubble Sort?
Bubble Sort is a comparison-based sorting algorithm.
4. Describe the primary benefit of Bubble Sort.
The greatest strength of Bubble Sort is that it is easy to understand and implement. It is easy to write and read.
5. What is the weakness of Bubble Sort?
The greatest weakness of Bubble Sort is that it has inefficient performance for sorting larger sets of data. When it sorts the large datasets, then it gives the high time complexity.
6. What is the best condition to use the Bubble Sort?
Bubble Sort is good for sorting data when there are small data sets or data is already sorted. It is good when we need simplicity instead of efficiency.
7. Can we use Bubble Sort to perform sorting of real-world applications?
Bubble Sort is rarely used in practical applications because of its inefficiency with large data sets. However, it can be used for instructional purposes and small data sets where we don't need efficiency.
8. Explain the process of Bubble Sort with an example.
The Bubble Sort algorithm is used to compare adjacent elements and swap them when they are unsorted. This is repeated until the list of elements becomes sorted. Let's understand the workings of the Bubble Sort With the following example:
Step 1. [5, 2, 9, 3] - Swapping the first two elements that are 5 and 2.
Step 2. [2, 5, 9, 3] - Now, it can't swap the 5 and 9 because 5 is shorter than 9.
Step 3. [2, 5, 9, 3] - Now it will switch to the third set, which is 9 and 3.
Step 4. [2, 5, 3, 9] - Now the first cycle is completed, and this cycle will run till no swaps are left in any cycle.
9. What is the process of optimizing the Bubble Sort?
We can optimize bubble sort by putting a flag to check if any swap was made during a pass through the array. If no swap was made, then the array is sorted, and the algorithm can be stopped early.
10. what is an "in-place" sorting algorithm?
An "in-place" sorting algorithm sorts the elements of an array without using extra space. It sorts the elements of an original array without using any additional data structures for storage.
11. What is the meaning of "Bubble" in Bubble Sort?
The word "Bubble" of Bubble Sort tells the sorting process of the list where small elements "bubble" up on the top of the list. This shows how small values easily come into place.
12. How to apply Bubble Sort on linked lists?
Bubble Sort can be used to sort linked lists. It is not the best algorithm for sorting and as well as big linked lists. We have better sorting algorithms for sorting this data structure.
13. How much stable algorithm is Bubble Sort?
Bubble Sort is known as a stable sorting algorithm. This is because it preserves the relative order of equal elements in the list. In this, two elements with the same value in the sorted array will retain their original order.
14. Why is the inner loop important in Bubble Sort?
The inner loop does the comparison and swapping of the next two elements to place the biggest unsorted element at the array end.
15. Can Bubble Sort sort non-integer data?
Yes, Bubble Sort can sort non-integer data, provided that a proper comparison function is set up for the data type.
16. What is the main advantage of Bubble Sort over other sorting algorithms?
Bubble Sort is simple to implement as well as to understand. Hence, it is excellent for educational needs. It's a good candidate for educational environments and small dataset collections where there is no stress about complexity based on its simple nature.
17. What is the result of sorting an already sorted array using a Bubble Sort algorithm?
When you have an already sorted array and apply a Bubble Sort algorithm to it, the Bubble Sort will perform a single pass and determine that the array has been sorted.
18. What are the pros and cons of Bubble Sort?
Advantages: Easy to implement and understand, ideal for educational use.
Disadvantages: Inefficient for big data, not stable, and high time complexity.
19. How can we handle a sorted array by Bubble Sort?
Yes, Bubble Sort can sort a partially sorted array more effectively by employing the "optimized Bubble Sort" method, which avoids unnecessary comparisons and swaps.
20. What is the compared efficiency with the bubble sort?
Bubble Sort is the least efficient among the sorting algorithms with a time complexity of O(N), whereas the time complexities of Merge Sort and Quick Sort are O(N log N).
21. What is the best case of Bubble Sort?
The best case for Bubble Sort is when the array is sorted. The time complexity of the best case is O(N). In this, the algorithm checks one time in the array where its no swaps at all.
22. What is the worst-case of Bubble Sort?
The worst-case scenario of Bubble Sort is when the array is sorted in reverse order. Time complexity in that case is O(N) because the algorithm has to do N swaps through the array, where each pass has up to N comparisons and swaps.
23. What is the effect of applying Bubble Sort recursively?
Bubble Sort is recursively called. Usually, it is not common practice. Using bubble sort recursively can head to function calling and the possibility of stack overflow for large arrays.
24. What is the process of treating the duplicate elements in Bubble Sort?
Bubble Sort is a stable sorting algorithm that keeps duplicate elements in the original relative order. If there are any two same elements, they would not be swapped, which will help to keep their relative position.
25. What is the function of the outer loop during Bubble Sort?
The outer loop of the Bubble Sort takes care of the passes through the array. Each pass shifts the largest element into its correct position, which is at the end of an array.