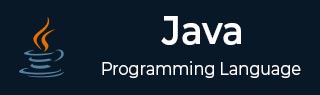
- Java - Home
- Java - Overview
- Java - History
- Java - Features
- Java Vs. C++
- JVM - Java Virtual Machine
- Java - JDK vs JRE vs JVM
- Java - Environment Setup
- Java - Hello World Program
- Java - Comments
- Java - Basic Syntax
- Java - Variables
- Java - Data Types
- Java - Type Casting
- Java - Unicode System
- Java - User Input
- Java - Date & Time
Java Operators
- Java - Operators
- Java - Arithmetic Operators
- Java - Assignment Operators
- Java - Relational Operators
- Java - Logical Operators
- Java - Bitwise Operators
- Java Operator Precedence & Associativity
Java Control Statements
- Java - Decision Making
- Java - If Else Statement
- Java - Switch Statement
- Java - Loop Control
- Java - For Loop
- Java - For-Each Loop
- Java - While Loop
- Java - Do While Loop
- Java - Break Statement
- Java - Continue Statement
Object Oriented Programming
- Java - OOPs Concepts
- Java - Object & Classes
- Java - Class Attributes
- Java - Class Methods
- Java - Methods
- Java - Variables Scope
- Java - Constructors
- Java - Access Modifiers
- Java - Inheritance
- Java - Aggregation
- Java - Polymorphism
- Java - Overriding
- Java - Method Overloading
- Java - Dynamic Binding
- Java - Static Binding
- Java - Instance Initializer Block
- Java - Abstraction
- Java - Encapsulation
- Java - Interfaces
- Java - Packages
- Java - Inner Classes
- Java - Static Class
- Java - Anonymous Class
- Java - Singleton Class
- Java - Wrapper Classes
- Java - Enums
- Java - Enum Constructor
- Java - Enum Strings
Java Built-in Classes
Java File Handling
- Java - Files
- Java - Create a File
- Java - Write to File
- Java - Read Files
- Java - Delete Files
- Java - Directories
- Java - I/O Streams
Java Error & Exceptions
- Java - Exceptions
- Java - try-catch Block
- Java - try-with-resources
- Java - Multi-catch Block
- Java - Nested try Block
- Java - Finally Block
- Java - throw Exception
- Java - Exception Propagation
- Java - Built-in Exceptions
- Java - Custom Exception
Java Multithreading
- Java - Multithreading
- Java - Thread Life Cycle
- Java - Creating a Thread
- Java - Starting a Thread
- Java - Joining Threads
- Java - Naming Thread
- Java - Thread Scheduler
- Java - Thread Pools
- Java - Main Thread
- Java - Thread Priority
- Java - Daemon Threads
- Java - Thread Group
- Java - Shutdown Hook
Java Synchronization
- Java - Synchronization
- Java - Block Synchronization
- Java - Static Synchronization
- Java - Inter-thread Communication
- Java - Thread Deadlock
- Java - Interrupting a Thread
- Java - Thread Control
- Java - Reentrant Monitor
Java Networking
- Java - Networking
- Java - Socket Programming
- Java - URL Processing
- Java - URL Class
- Java - URLConnection Class
- Java - HttpURLConnection Class
- Java - Socket Class
- Java - Generics
Java Collections
Java Interfaces
- Java - List Interface
- Java - Queue Interface
- Java - Map Interface
- Java - SortedMap Interface
- Java - Set Interface
- Java - SortedSet Interface
Java Data Structures
Java Collections Algorithms
Advanced Java
- Java - Command-Line Arguments
- Java - Lambda Expressions
- Java - Sending Email
- Java - Applet Basics
- Java - Javadoc Comments
- Java - Autoboxing and Unboxing
- Java - File Mismatch Method
- Java - REPL (JShell)
- Java - Multi-Release Jar Files
- Java - Private Interface Methods
- Java - Inner Class Diamond Operator
- Java - Multiresolution Image API
- Java - Collection Factory Methods
- Java - Module System
- Java - Nashorn JavaScript
- Java - Optional Class
- Java - Method References
- Java - Functional Interfaces
- Java - Default Methods
- Java - Base64 Encode Decode
- Java - Switch Expressions
- Java - Teeing Collectors
- Java - Microbenchmark
- Java - Text Blocks
- Java - Dynamic CDS archive
- Java - Z Garbage Collector (ZGC)
- Java - Null Pointer Exception
- Java - Packaging Tools
- Java - Sealed Classes
- Java - Record Classes
- Java - Hidden Classes
- Java - Pattern Matching
- Java - Compact Number Formatting
- Java - Garbage Collection
- Java - JIT Compiler
Java Miscellaneous
- Java - Recursion
- Java - Regular Expressions
- Java - Serialization
- Java - Strings
- Java - Process API Improvements
- Java - Stream API Improvements
- Java - Enhanced @Deprecated Annotation
- Java - CompletableFuture API Improvements
- Java - Streams
- Java - Datetime Api
- Java 8 - New Features
- Java 9 - New Features
- Java 10 - New Features
- Java 11 - New Features
- Java 12 - New Features
- Java 13 - New Features
- Java 14 - New Features
- Java 15 - New Features
- Java 16 - New Features
Java APIs & Frameworks
Java Class References
- Java - Scanner
- Java - Arrays
- Java - Strings
- Java - Date
- Java - ArrayList
- Java - Vector
- Java - Stack
- Java - PriorityQueue
- Java - LinkedList
- Java - ArrayDeque
- Java - HashMap
- Java - LinkedHashMap
- Java - WeakHashMap
- Java - EnumMap
- Java - TreeMap
- Java - IdentityHashMap
- Java - HashSet
- Java - EnumSet
- Java - LinkedHashSet
- Java - TreeSet
- Java - BitSet
- Java - Dictionary
- Java - Hashtable
- Java - Properties
- Java - Collection
- Java - Array
Java Useful Resources
Java - Relational Operators
Java relational operators are used to compare two values. These operators return a boolean result: true
if the condition is met and false
otherwise. Relational operators are commonly used in decision-making statements like if
conditions and loops.
Java provides several relational operators that can be applied to primitive data types such as int
, float
, double
, and char
. These operators help determine equality, inequality, and relative comparison between values.
List of Java Relational Operators
The following table lists the relational operators in Java:
Operator | Description | Example |
---|---|---|
== (equal to) | Checks if two values are equal. | (A == B) returns false if A is not equal to B. |
!= (not equal to) | Checks if two values are not equal. | (A != B) returns true if A is not equal to B. |
> (greater than) | Checks if the left operand is greater than the right operand. | (A > B) returns true if A is greater than B. |
< (less than) | Checks if the left operand is smaller than the right operand. | (A < B) returns true if A is less than B. |
>= (greater than or equal to) | Checks if the left operand is greater than or equal to the right operand. | (A >= B) returns true if A is greater than or equal to B. |
<= (less than or equal to) | Checks if the left operand is smaller than or equal to the right operand. | (A <= B) returns true if A is less than or equal to B. |
The following programs are simple examples which demonstrate the relational operators. Copy and paste the following Java programs as Test.java file, and compile and run the programs −
Example 1
In this example, we're creating two variables a and b and using relational operators. We've performed equality and non-equality checks and printed the results.
public class Test { public static void main(String args[]) { int a = 10; int b = 20; System.out.println("a == b = " + (a == b) ); System.out.println("a != b = " + (a != b) ); } }
Output
a == b = false a != b = true
Example 2
In this example, we're creating two variables a and b and using relational operators. We've performed greater than and less than checks and printed the results.
public class Test { public static void main(String args[]) { int a = 10; int b = 20; System.out.println("a > b = " + (a > b) ); System.out.println("a < b = " + (a < b) ); } }
Output
a > b = false a < b = true
Example 3
In this example, we're creating two variables a and b and using relational operators. We've performed greater than or equal to and less than or equal to checks and printed the results.
public class Test { public static void main(String args[]) { int a = 10; int b = 20; System.out.println("b >= a = " + (b >= a) ); System.out.println("b <= a = " + (b <= a) ); } }
Output
b >= a = true b <= a = false