API Docs Unified API v2.0
API v1.0
We also offer another API version with endpoints that are easier to integrate. If you're a beginner in implementing API's you can switch to our v1.0 endpoint.
Query by first name
This is the easiest way to query a gender by a name. Each response is JSON encoded:
Post
Required Requests: 1
URL | https://gender-api.com/v2/gender | ||||||||||||||||||||||||
Headers | Content-Type: application/json Authorization: Bearer <your authorization token> | ||||||||||||||||||||||||
JSON Payload |
|
Examples
Query by first name only
{"first_name":"Sandra"}
Query by first name and country code
{"first_name":"Sandra","country":"US"}
Query by first name and browser locale
{"first_name":"Sandra","locale":"en_US"}
Query by first name and use an IP address for localization
{"first_name":"Sandra","ip":"54.201.16.177"}
Query multiple names in a single request
[{"first_name":"Sandra","country":"US"},{"first_name":"Jason","country":"US"}]
Response
{ "input": { "first_name": "Sandra" }, "details": { "credits_used": 1, "samples": 464, "country": null, "first_name_sanitized": "sandra", "duration": "436ms" }, "result_found": true, "first_name": "Sandra", "probability": 0.85, "gender": "female" }
Field | Type | Description |
---|---|---|
input | object | The submitted payload |
details.credits_used | int | The amount of requests used for this query |
details.samples | int | Number of records found in our database which match your request |
details.country | string | The country we found |
details.first_name_sanitized | string | The name after we applied our normalizer to it |
details.duration | string | Time the server needed to process the request |
result_found | bool | True if we were able to query a gender for the given name |
first_name | string | The first name we used for genderization |
probability | float | This value (between 0 and 1) determines the reliability of our database. A value of 1 means that the results on your gender request are 100% accurate. |
gender | string | Possible values: male, female, unknown |
Query by full name - SPLIT FIRST AND LAST NAME
If you use a combined field for first and last name on your page, use this API to extract the parts:
Post
Required Requests: 1
URL | https://gender-api.com/v2/gender | ||||||||||||||||||||||||
Headers | Content-Type: application/json Authorization: Bearer <your authorization token> | ||||||||||||||||||||||||
JSON Payload |
|
Examples
Query by full name only
{"full_name":"Theresa Miller"}
Query by full name and country code
{"full_name":"Theresa Miller","country":"US"}
Query by full name and browser locale
{"full_name":"Thomas Johnson","locale":"en_US"}
Query by full name and use an IP address for localization
{"full_name":"Markus Stefan NonExistingLastName","ip":"54.201.16.177"}
Query multiple names in a single request
[{"full_name":"Theresa Miller"},{"full_name":"Thomas Johnson","country":"US"}]
Response
{ "input": { "full_name": "Theresa Miller" }, "details": { "credits_used": 1, "duration": "33ms", "samples": 8961, "country": null, "first_name_sanitized": "theresa" }, "result_found": true, "last_name": "Miller", "first_name": "Theresa", "probability": 0.98, "gender": "female" }
Field | Type | Description |
---|---|---|
input | object | The submitted payload |
details.credits_used | int | The amount of requests used for this query |
details.samples | int | Number of records found in our database which match your request |
details.country | string | The country we found |
details.full_name_sanitized | int | The name after we applied our normalizer to it |
details.duration | string | Time the server needed to process the request |
result_found | bool | True if we were able to query a gender for the given name |
full_name | string | The full name we used for genderization |
probability | float | This value (between 0 and 1) determines the reliability of our database. A value of 1 means that the results on your gender request are 100% accurate. |
gender | string | Possible values: male, female, unknown |
Query by email address
You can also query a gender using an email address containing a email address:
Post
Required Requests: 1
URL | https://gender-api.com/v2/gender | ||||||||||||||||||||||||
Headers | Content-Type: application/json Authorization: Bearer <your authorization token> | ||||||||||||||||||||||||
JSON Payload |
|
Examples
Query by email address only
{"email":"theresa.miller14@gmail.com"}
Query by email address and country code
{"email":"thomas.clarks@hotmail.com","country":"US"}
Query by email address and browser locale
{"email":"Thomas.j32@live.com","locale":"en_US"}
Query by email address and use an IP address for localization
{"email":"thomasfromnewyork@gmail.com","ip":"54.201.16.177"}
Query multiple names in a single request
[{"email":"thomas.clarks@hotmail.com","country":"US"},{"email":"theresa.miller14@gmail.com"}]
Response
{ "input": { "email": "theresa.miller14@gmail.com" }, "details": { "credits_used": 1, "duration": "12ms", "samples": 8961, "country": null, "first_name_sanitized": "theresa" }, "result_found": true, "last_name": "Miller", "first_name": "Theresa", "probability": 0.98, "gender": "female" }
Field | Type | Description |
---|---|---|
input | object | The submitted payload |
details.credits_used | int | The amount of requests used for this query |
details.samples | int | Number of records found in our database which match your request |
details.country | string | The country we found |
details.email_sanitized | int | The name after we applied our normalizer to it |
details.duration | string | Time the server needed to process the request |
result_found | bool | True if we were able to query a gender for the given name |
string | The email address we used for genderization | |
probability | float | This value (between 0 and 1) determines the reliability of our database. A value of 1 means that the results on your gender request are 100% accurate. |
gender | string | Possible values: male, female, unknown |
Get Country Of Origin
Get a name's country of origin:
Post
Required Requests: 2
URL | https://gender-api.com/v2/country-of-origin | ||||||||||||||||||||
Headers | Content-Type: application/json Authorization: Bearer <your authorization token> | ||||||||||||||||||||
JSON Payload |
|
Examples
Query by first name
{"first_name":"Johann"}
Query by full name
{"full_name":"Theresa Miller"}
Query by email address
{"full_name":"sophia5342@gmail.com"}
Response
{ "input": { "first_name": "Johann", "id": 12 }, "details": { "credits_used": 2, "duration": "414ms", "samples": 890, "country": null, "first_name_sanitized": "johann" }, "result_found": true, "country_of_origin": [ { "country_name": "Germany", "country": "DE", "probability": 0.52, "continental_region": "Europe", "statistical_region": "Western Europe" }, { "country_name": "Austria", "country": "AT", "probability": 0.48, "continental_region": "Europe", "statistical_region": "Western Europe" } ], "first_name": "Johann", "probability": 0.9, "gender": "male", "language_of_origin": "Germanic", "meaning": "Johann is the German variation of the name John, which means 'God is gracious'. It was frequently used in the Middle Ages across Europe, particularly as a name for religious figures.", "ethnicity": { "id": "GERMANIC", "name": "Germanic (German, Austrian, Swiss)", "distribution": [ { "id": "GERMANIC", "name": "Germanic (German, Austrian, Swiss)", "percentage": 90 }, { "id": "DUTCH", "name": "Dutch", "percentage": 5 }, { "id": "FRENCH", "name": "French", "percentage": 5 } ] } }
Field | Type | Description |
---|---|---|
input | object | The submitted payload |
details.credits_used | int | The amount of requests used for this query |
details.samples | int | Number of records found in our database which match your request |
details.country | string | The country we found |
details.first_name_sanitized | string | The name after we applied our normalizer to it |
details.duration | string | Time the server needed to process the request |
result_found | bool | True if we were able to query a gender for the given name |
country_of_origin_map_url | string | URL to an interactive map about this name |
first_name | string | The first name we used for genderization |
probability | float | This value (between 0 and 1) determines the reliability of our database. A value of 1 means that the results on your gender request are 100% accurate |
gender | string | Possible values: male, female, unknown |
country_of_origin | object | Top 25 countries of origin. See "Complete List Of Possible Countries" for a list of supported countries. Every record contains the fields country_name, country, probability, continental_region and statistical_region |
language_of_origin | string | The origin language of this name |
meaning | string | A short description of the meaning of this name |
ethnicity | object | The ethnicity field provides details on the primary ethnic group associated with the name, along how the name is distributed across various ethnic groups |
ethnicity.id | string | The id field contains an identifier for the determined main ethnic group. See "Complete List Of Possible Ethnic Groups" for a full list of possible ethnic groups |
ethnicity.name | string | The name field contains the name of the determined main ethnic group. See "Complete List Of Possible Ethnic Groups" for a full list of possible ethnic groups |
ethnicity.distribution | array | The Distribution field contains information about the proportion of the name within different ethnic groups. Each array entry contains the fields id (string), name (string) and percentage (float). See "Complete List Of Possible Ethnic Groups" for a full list of possible values |
Screenshot Interactive Map
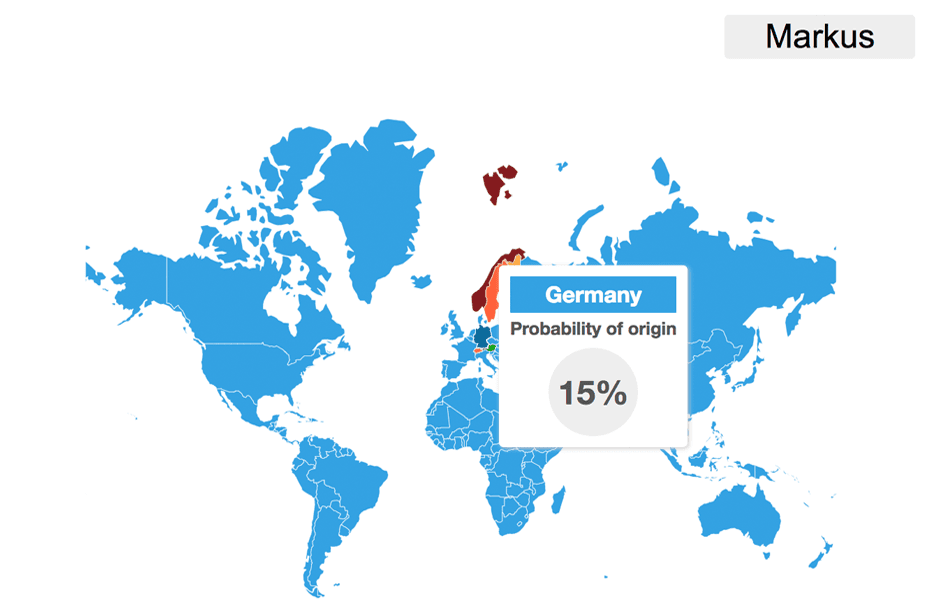
Complete List Of Possible Countries
Country Code | Country Name | Statistical Region | Continental Region |
---|---|---|---|
CN | China | Eastern Asia | Asia |
IN | India | Southern Asia | Asia |
US | United States | Northern America | Americas |
ID | Indonesia | South-eastern Asia | Asia |
BR | Brazil | South America | Americas |
PK | Pakistan | Southern Asia | Asia |
NG | Nigeria | Western Africa | Africa |
BD | Bangladesh | Southern Asia | Asia |
RU | Russia | Eastern Europe | Europe |
MX | Mexico | Central America | Americas |
JP | Japan | Eastern Asia | Asia |
ET | Ethiopia | Eastern Africa | Africa |
PH | Philippines | South-eastern Asia | Asia |
EG | Egypt | Northern Africa | Africa |
VN | Vietnam | South-eastern Asia | Asia |
DE | Germany | Western Europe | Europe |
CD | Democratic Republic of the Congo | Middle Africa | Africa |
IR | Iran | Southern Asia | Asia |
TR | Turkey | Western Asia | Asia |
TH | Thailand | South-eastern Asia | Asia |
GB | United Kingdom | Northern Europe | Europe |
FR | France | Western Europe | Europe |
IT | Italy | Southern Europe | Europe |
TZ | Tanzania | Eastern Africa | Africa |
ZA | South Africa | Southern Africa | Africa |
MM | Myanmar | South-eastern Asia | Asia |
KR | South Korea | Eastern Asia | Asia |
CO | Colombia | South America | Americas |
KE | Kenya | Eastern Africa | Africa |
ES | Spain | Southern Europe | Europe |
AR | Argentina | South America | Americas |
UA | Ukraine | Eastern Europe | Europe |
UG | Uganda | Eastern Africa | Africa |
DZ | Algeria | Northern Africa | Africa |
SD | Sudan | Northern Africa | Africa |
IQ | Iraq | Western Asia | Asia |
PL | Poland | Eastern Europe | Europe |
CA | Canada | Northern America | Americas |
MA | Morocco | Northern Africa | Africa |
AF | Afghanistan | Southern Asia | Asia |
SA | Saudi Arabia | Western Asia | Asia |
PE | Peru | South America | Americas |
VE | Venezuela | South America | Americas |
UZ | Uzbekistan | Central Asia | Asia |
MY | Malaysia | South-eastern Asia | Asia |
AO | Angola | Middle Africa | Africa |
MZ | Mozambique | Eastern Africa | Africa |
NP | Nepal | Southern Asia | Asia |
GH | Ghana | Western Africa | Africa |
YE | Yemen | Western Asia | Asia |
MG | Madagascar | Eastern Africa | Africa |
KP | North Korea | Eastern Asia | Asia |
AU | Australia | AustraliaandNew Zealand | Oceania |
CI | Ivory Coast | Western Africa | Africa |
CM | Cameroon | Middle Africa | Africa |
TW | Taiwan | Eastern Asia | Asia |
NE | Niger | Western Africa | Africa |
LK | Sri Lanka | Southern Asia | Asia |
RO | Romania | Eastern Europe | Europe |
BF | Burkina Faso | Western Africa | Africa |
MW | Malawi | Eastern Africa | Africa |
ML | Mali | Western Africa | Africa |
SY | Syria | Western Asia | Asia |
KZ | Kazakhstan | Central Asia | Asia |
CL | Chile | South America | Americas |
ZM | Zambia | Eastern Africa | Africa |
NL | Netherlands | Western Europe | Europe |
GT | Guatemala | Central America | Americas |
EC | Ecuador | South America | Americas |
ZW | Zimbabwe | Eastern Africa | Africa |
KH | Cambodia | South-eastern Asia | Asia |
SN | Senegal | Western Africa | Africa |
TD | Chad | Middle Africa | Africa |
SO | Somalia | Eastern Africa | Africa |
GN | Guinea | Western Africa | Africa |
SD | Sudan | Northern Africa | Africa |
RW | Rwanda | Eastern Africa | Africa |
TN | Tunisia | Northern Africa | Africa |
CU | Cuba | Caribbean | Americas |
BE | Belgium | Western Europe | Europe |
BJ | Benin | Western Africa | Africa |
GR | Greece | Southern Europe | Europe |
BO | Bolivia | South America | Americas |
HT | Haiti | Caribbean | Americas |
BI | Burundi | Eastern Africa | Africa |
DO | Dominican Republic | Caribbean | Americas |
CZ | Czech Republic | Eastern Europe | Europe |
PT | Portugal | Southern Europe | Europe |
SE | Sweden | Northern Europe | Europe |
AZ | Azerbaijan | Western Asia | Asia |
HU | Hungary | Eastern Europe | Europe |
JO | Jordan | Western Asia | Asia |
BY | Belarus | Eastern Europe | Europe |
AE | United Arab Emirates | Western Asia | Asia |
HN | Honduras | Central America | Americas |
TJ | Tajikistan | Central Asia | Asia |
RS | Serbia | Southern Europe | Europe |
AT | Austria | Western Europe | Europe |
CH | Switzerland | Western Europe | Europe |
IL | Israel | Western Asia | Asia |
PG | Papua New Guinea | Melanesia | Oceania |
TG | Togo | Western Africa | Africa |
SL | Sierra Leone | Western Africa | Africa |
HK | Hong Kong | Eastern Asia | Asia |
BG | Bulgaria | Eastern Europe | Europe |
LA | Laos | South-eastern Asia | Asia |
PY | Paraguay | South America | Americas |
SV | El Salvador | Central America | Americas |
LY | Libya | Northern Africa | Africa |
NI | Nicaragua | Central America | Americas |
LB | Lebanon | Western Asia | Asia |
KG | Kyrgyzstan | Central Asia | Asia |
TM | Turkmenistan | Central Asia | Asia |
DK | Denmark | Northern Europe | Europe |
SG | Singapore | South-eastern Asia | Asia |
FI | Finland | Northern Europe | Europe |
SK | Slovakia | Eastern Europe | Europe |
NO | Norway | Northern Europe | Europe |
CG | Congo | Middle Africa | Africa |
ER | Eritrea | Eastern Africa | Africa |
PS | Palestine | Western Asia | Asia |
CR | Costa Rica | Central America | Americas |
IE | Ireland | Northern Europe | Europe |
LR | Liberia | Western Africa | Africa |
NZ | New Zealand | AustraliaandNew Zealand | Oceania |
CF | Central African Republic | Middle Africa | Africa |
OM | Oman | Western Asia | Asia |
MR | Mauritania | Western Africa | Africa |
HR | Croatia | Southern Europe | Europe |
KW | Kuwait | Western Asia | Asia |
PA | Panama | Central America | Americas |
MD | Moldova | Eastern Europe | Europe |
GE | Georgia | Western Asia | Asia |
PR | Puerto Rico | Caribbean | Americas |
BA | Bosnia and Herzegovina | Southern Europe | Europe |
UY | Uruguay | South America | Americas |
MN | Mongolia | Eastern Asia | Asia |
AM | Armenia | Western Asia | Asia |
AL | Albania | Southern Europe | Europe |
JM | Jamaica | Caribbean | Americas |
LT | Lithuania | Northern Europe | Europe |
QA | Qatar | Western Asia | Asia |
NA | Namibia | Southern Africa | Africa |
BW | Botswana | Southern Africa | Africa |
LS | Lesotho | Southern Africa | Africa |
GM | The Gambia | Western Africa | Africa |
MK | Republic of Macedonia | Southern Europe | Europe |
SI | Slovenia | Southern Europe | Europe |
GA | Gabon | Middle Africa | Africa |
LV | Latvia | Northern Europe | Europe |
GW | Guinea-Bissau | Western Africa | Africa |
BH | Bahrain | Western Asia | Asia |
TT | Trinidad and Tobago | Caribbean | Americas |
SZ | Swaziland | Southern Africa | Africa |
EE | Estonia | Northern Europe | Europe |
TL | East Timor | South-eastern Asia | Asia |
GQ | Equatorial Guinea | Middle Africa | Africa |
MU | Mauritius | Eastern Africa | Africa |
CY | Cyprus | Western Asia | Asia |
DJ | Djibouti | Eastern Africa | Africa |
FJ | Fiji | Melanesia | Oceania |
RE | Réunion | Eastern Africa | Africa |
KM | Comoros | Eastern Africa | Africa |
BT | Bhutan | Southern Asia | Asia |
GY | Guyana | South America | Americas |
ME | Montenegro | Southern Europe | Europe |
MO | Macau | Eastern Asia | Asia |
SB | Solomon Islands | Melanesia | Oceania |
LU | Luxembourg | Western Europe | Europe |
SR | Suriname | South America | Americas |
EH | Western Sahara | Northern Africa | Africa |
CV | Cape Verde | Western Africa | Africa |
GP | Guadeloupe | Caribbean | Americas |
MV | Maldives | Southern Asia | Asia |
MT | Malta | Southern Europe | Europe |
BN | Brunei | South-eastern Asia | Asia |
BS | Bahamas | Caribbean | Americas |
MQ | Martinique | Caribbean | Americas |
BZ | Belize | Central America | Americas |
IS | Iceland | Northern Europe | Europe |
BB | Barbados | Caribbean | Americas |
PF | French Polynesia | Polynesia | Oceania |
GF | French Guiana | South America | Americas |
NC | New Caledonia | Melanesia | Oceania |
VU | Vanuatu | Melanesia | Oceania |
YT | Mayotte | Eastern Africa | Africa |
ST | Sao Tome and Principe | Middle Africa | Africa |
WS | Samoa | Polynesia | Oceania |
LC | Saint Lucia | Caribbean | Americas |
JE | Guernsey and Jersey | Northern Europe | Europe |
GU | Guam | Micronesia | Oceania |
CW | Curaçao | Caribbean | Americas |
KI | Kiribati | Micronesia | Oceania |
VC | Saint Vincent and the Grenadines | Caribbean | Americas |
TO | Tonga | Polynesia | Oceania |
GD | Grenada | Caribbean | Americas |
FM | Federated States of Micronesia | Micronesia | Oceania |
AW | Aruba | Caribbean | Americas |
VI | United States Virgin Islands | Caribbean | Americas |
AG | Antigua and Barbuda | Caribbean | Americas |
SC | Seychelles | Eastern Africa | Africa |
IM | Isle of Man | Northern Europe | Europe |
AD | Andorra | Southern Europe | Europe |
DM | Dominica | Caribbean | Americas |
KY | Cayman Islands | Caribbean | Americas |
BM | Bermuda | Northern America | Americas |
GL | Greenland | Northern America | Americas |
AS | American Samoa | Polynesia | Oceania |
KN | Saint Kitts and Nevis | Caribbean | Americas |
MP | Northern Mariana Islands | Micronesia | Oceania |
MH | Marshall Islands | Micronesia | Oceania |
FO | Faroe Islands | Northern Europe | Europe |
SX | Sint Maarten | Caribbean | Americas |
MC | Monaco | Western Europe | Europe |
LI | Liechtenstein | Western Europe | Europe |
TC | Turks and Caicos Islands | Caribbean | Americas |
GI | Gibraltar | Southern Europe | Europe |
SM | San Marino | Southern Europe | Europe |
VG | British Virgin Islands | Caribbean | Americas |
BQ | Caribbean Netherlands | Caribbean | Americas |
PW | Palau | Micronesia | Oceania |
CK | Cook Islands | Polynesia | Oceania |
AI | Anguilla | Caribbean | Americas |
WF | Wallis and Futuna | Polynesia | Oceania |
NR | Nauru | Micronesia | Oceania |
TV | Tuvalu | Polynesia | Oceania |
PM | Saint Pierre and Miquelon | Northern America | Americas |
MS | Montserrat | Caribbean | Americas |
SH | Saint Helena Ascension and Tristan da Cunha | Western Africa | Africa |
FK | Falkland Islands | South America | Americas |
NU | Niue | Polynesia | Oceania |
TK | Tokelau | Polynesia | Oceania |
VA | Vatican City | Southern Europe | Europe |
Complete List Of Possible Ethnic Groups
ID | Name |
---|---|
GERMANIC | Germanic (German, Austrian, Swiss) |
SCANDINAVIAN | Scandinavian (Swedish, Norwegian, Danish, Finnish) |
ENGLISH | English |
DUTCH | Dutch |
HUNGARIAN | Hungarian |
ESTONIAN | Estonian |
LATVIAN | Latvian |
LITHUANIAN | Lithuanian |
SLOVENIAN | Slovenian |
CROATIAN | Croatian |
SERBIAN | Serbian |
BOSNIAN | Bosnian |
MACEDONIAN | Macedonian |
BULGARIAN | Bulgarian |
ROMANIAN | Romanian |
POLISH | Polish |
SLOVAK | Slovak |
CZECH | Czech |
RUSSIAN | Russian |
UKRAINIAN | Ukrainian |
BELARUSIAN | Belarusian |
ICELANDIC | Icelandic |
FAROESE | Faroese |
SCOTTISH | Scottish |
IRISH | Irish |
WELSH | Welsh |
ITALIAN | Italian |
SPANISH | Spanish |
PORTUGUESE | Portuguese |
FRENCH | French |
GREEK | Greek |
TURKISH | Turkish |
ARMENIAN | Armenian |
GEORGIAN | Georgian |
ALBANIAN | Albanian |
KURDISH | Kurdish |
PERSIAN | Persian |
ARAB | Arab (Various Arabic-speaking countries) |
HEBREW | Hebrew |
INDIAN | Indian |
PAKISTANI | Pakistani |
BANGLADESHI | Bangladeshi |
SRI_LANKAN | Sri Lankan |
NEPALI | Nepali |
BHUTANESE | Bhutanese |
TIBETAN | Tibetan |
CHINESE | Chinese |
JAPANESE | Japanese |
KOREAN | Korean |
VIETNAMESE | Vietnamese |
THAI | Thai |
MALAYSIAN | Malaysian |
INDONESIAN | Indonesian |
FILIPINO | Filipino |
AUSTRALIAN_ABORIGINAL | Australian Aboriginal |
MAORI | Maori (New Zealand) |
NATIVE_AMERICAN | Native American (various tribes in North and South America) |
INUIT | Inuit (Arctic regions) |
AFRICAN | African ethnic groups (various countries and tribes) |
PACIFIC_ISLANDER | Pacific Islander (various islands and cultures) |
Get Stats
With this call you can pull some stats on your account:
Get
URL | https://gender-api.com/v2/statistic |
Headers | Content-Type: application/json Authorization: Bearer <your authorization token> |
Response
{ "is_limit_reached": false, "remaining_credits": 24999, "details": { "credits_used": 0, "duration": "83ms" }, "usage_last_month": { "date": "2018-03", "credits_used": 25 } }
Field | Type | Description |
---|---|---|
is_limit_reached | bool | Returns true if there are no more requests left |
remaining_credits | int | Number of requests left |
details.credits_used | int | The amount of requests used for this query |
details.duration | string | Time the server needed to process the request |
usage_last_month.date | string | Last month's date |
usage_last_month.credits_used | int | Requests that you have used over the last month |
Error Codes
Status | endpoint-not-found |
HTTP Status Code | 404 |
Description | API endpoint not found |
Status | limit-exceeded |
HTTP Status Code | 401 |
Description | Limit exceeded. Thank you for using our service. Please consider to buy more requests. |
Status | parameter-first-name-invalid |
HTTP Status Code | 400 |
Description | The "first_name" parameter is invalid. The parameter can only be a string with a length from 1 to 50 chars. |
Status | parameter-full-name-invalid |
HTTP Status Code | 400 |
Description | The "full_name" parameter is invalid. The parameter can only be a string with a length from 1 to 100 chars. |
Status | parameter-email-invalid |
HTTP Status Code | 400 |
Description | The "email" parameter is invalid. The parameter can only be a string with a length from 3 to 100 chars and must be a valid email address. |
Status | key-parameter-missing |
HTTP Status Code | 400 |
Description | The "key" parameter is not set. Please create an account to fetch an API key. |
Status | invalid-key |
HTTP Status Code | 400 |
Description | Invalid API key. You can find your personal API key in your account. |
Status | invalid-country-code |
HTTP Status Code | 400 |
Description | The given country code is not valid. You can find a list of valid country codes in our API docs. |
Status | invalid-ip-address |
HTTP Status Code | 400 |
Description | The given ip address is not valid. Please provide either a valid IPv4 or an IPv6 address. |
Status | generic-error |
HTTP Status Code | 400 |
Description |
Status | invalid-json |
HTTP Status Code | 400 |
Description |
Status | invalid-locale |
HTTP Status Code | 400 |
Description | The given locale is not valid. You can find a list of valid country codes in our API docs. |
Status | invalid-id |
HTTP Status Code | 400 |
Description | The given id is not valid. An id can be either an integer or a string with 50 chars at max. |
Status | mandatory-parameter-missing |
HTTP Status Code | 400 |
Description | Mandatory parameter missing. Either first_name, full_name or email must be set. |
Status | content-type-missing |
HTTP Status Code | 400 |
Description | Content-Type missing. Please add a "Content-Type: application/json" to your API call. |
Status | missing-json |
HTTP Status Code | 400 |
Description | We weren't able to find a json body in your API call. Please make sure the body is sent with a POST request to our API endpoint. |
Status | too-many-queries |
HTTP Status Code | 400 |
Description | Too many queries in a single API call. |
Status | too-many-queries-api-key |
HTTP Status Code | 400 |
Description | Too many queries in a single API call. Your API key is limited to 3 lookups per request. |
Status | authorization-header-missing |
HTTP Status Code | 400 |
Description | Your query misses the "Authorization" header with the bearer token. |
Status | invalid-auth-token |
HTTP Status Code | 400 |
Description | Invalid authentication token provided. Please log in to your account to find a list of valid tokens or to create a new one. |
Status | email-invalid |
HTTP Status Code | 400 |
Description | Invalid or empty email address given. |
Status | password-invalid |
HTTP Status Code | 400 |
Description | Invalid credentials. Login failed. Please also check your account that third-party app access is not restricted.: https://gender-api.com/de/account/password |
Status | label-invalid |
HTTP Status Code | 400 |
Description | Invalid label given. A label must be a string between 2 and 30 chars. |
Status | email-account-not-found |
HTTP Status Code | 400 |
Description | No Gender-API.com account found. Please create a free account first, to sign-in with Google or try to login with an existing username or password. |
Status | invalid-list-of-names |
HTTP Status Code | 400 |
Description | Invalid list of names. Expected array of strings. |
This product includes GeoLite2 data created by MaxMind, available from http://www.maxmind.com.